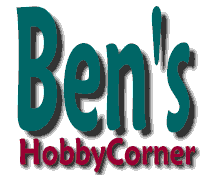
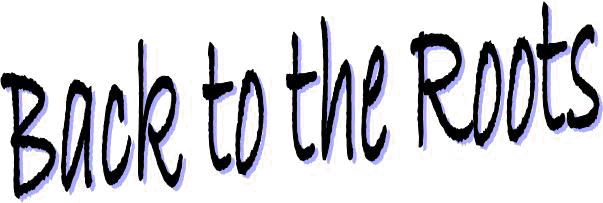 |
Here you can find
a step-by-step tutorial about the Bascom-AVR code to drive an AVR
and an WIZ810MJ Ethernet controller.

DHCP and this time on a Arduino Ethernetshield
New on
the long and short board:
UDP-switch that can be controlled by a iPhone app
UDP_remote
New on
the Red Bokito:
Email-switcher with confirmation-mail
There is
now also a version running on the Wiz812MJ
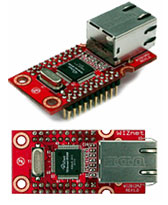
This tutorial is based on my own hardware
Step
1. Getting the hardware...
|
You
can cut and paste all green parts of the next text and place it into
the Bascom-AVR IDE.

This is the small sandwichboard.
There must be about 200 running at the moment.

The long board,
module, relays, RS232, all on
one single PCB
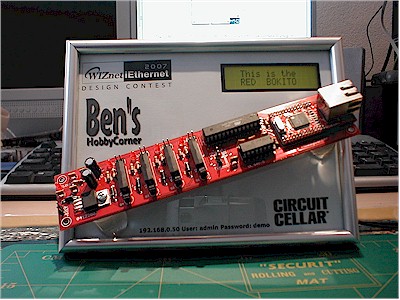
Here the board on the
Embedded Systems Conference in San Jose

The long bare PCBs

The small board with
the separate relay and RS232 board.
In this tutorial I use
both, the long and the small board.
Here
the schematics:
and
here another part
The Atmega168 microcontroller that is
used on the above board has some fuse bits. Care should
be taken while programming these fuse bits.
First, start with a small description
what you are planning to do with date and/or version-number.
'-----------------------------------------------------------
' Atmega168 and WIZ810MJ
'-----------------------------------------------------------
' Version 1.0 - July 2008
Make a small note
what you did with the fuse bits of the microcontroller
For this program the following fuse bits
should be set:
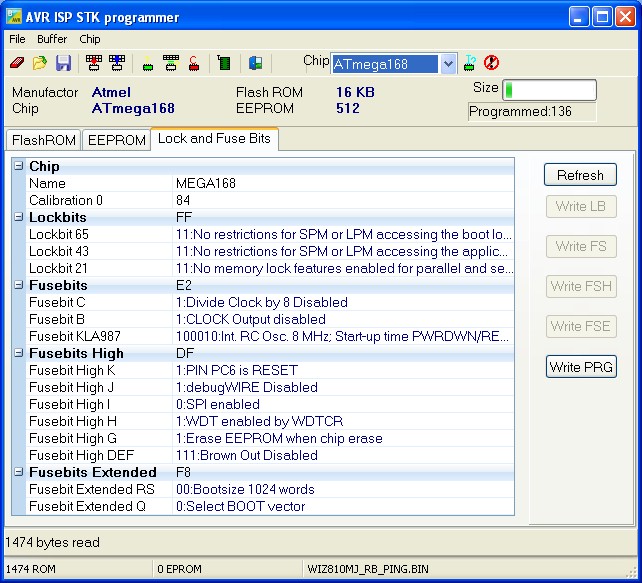
$crystal = 8000000
$regfile = "M168def.dat"
$hwstack = 64
$swstack = 64
$framesize = 64
$crystal is used to
give the MHz or Megacycles you are using.
$regfile is used to have Bascom-AVR use the right registers for the
right microcontroller. In this case we are using a Atmega168.
|
Step
2: Preparing the SPI-port and Chip-select-pin |
The next piece of code is to configure two output pins.
On the long board reset of the
WIZ810MJ is PORTC.0
On the sandwich board reset of the WIZ810MJ is PORTD.3
And the relays are on different pins
Long board:
Relais 1 on PORTC.1
Relais 2 on PORTC.2
Relais 3 on PORTC.3
Relais 4 on PORTC.4
Sandwichboard:
Relais 1 on PORTD.4
Relais 2 on PORTD.5
Relais 3 on PORTD.6
Relais 4 on PORTD.7
To make your code a
bit more readable, you can use aliases. To create one code for both
boards you can use #IF #ENDIF combinations.
' 0 = long board
' 1 = sandwichboard
Const Board = 1
'Used Wiz5100 ports and pins
Wiz5100_cs Alias Portb.2
'Chipselect WIZ810MJ
#if Board
'sandwichboard
Wiz5100_res Alias Portd.3
'reset of WIZ810MJ
'Other used ports and pins
Relais1 Alias Portd.4
Relais2 Alias Portd.5
Relais3 Alias Portd.6
Relais4 Alias Portd.7
#elseif
Wiz5100_res Alias Portc.0
'long board
Relais1 Alias Portc.1
Relais2 Alias Portc.2
Relais3 Alias Portc.3
Relais4 Alias Portc.4
#endif
Configure them as output
'Used ports and pins
Config Wiz5100_cs = Output
Config Wiz5100_res = Output
The WIZ810MJ is a stand-alone Ethernet
controller with an industry standard Serial Peripheral Interface (SPI).
It is designed to serve as an Ethernet network interface for any
controller equipped with SPI. With a single Config SPI command
Bascom-AVR configures the complete SPI-bus. See the Help for Bascom-AVR
for every single parameter of the Config SPI command.
'Configuration
of the SPI-bus
Config Spi = Hard , Interrupt = Off , Data Order = Msb , Master = Yes ,
Polarity = Low , Phase = 0 , Clockrate = 4 , Noss = 0
'init the spi pins
Spiinit
This is all you need to communicate
with the WIZ810MJ Ethernet module. |
Step
3: Believe it or not.... |
1474 bytes!!
1474 bytes, that
is all you need to get PING working on the WIZ810MJ-module.
'--------------------------------------------------------------
' Atmega168 and WIZ810MJ All you need to get a PING working
'--------------------------------------------------------------
$regfile = "m168def.dat"
$crystal = 8000000
$hwstack = 64
$swstack = 64
$framesize = 64
'Common registers
'Mode register
Const W5100_mr = &H0000
'Gateway address registers
Const W5100_gar0 = &H0001
Const W5100_gar1 = &H0002
Const W5100_gar2 = &H0003
Const W5100_gar3 = &H0004
'Subnet mask Address registers
Const W5100_subr0 = &H0005
Const W5100_subr1 = &H0006
Const W5100_subr2 = &H0007
Const W5100_subr3 = &H0008
'Source Hardware Address registers
Const W5100_shar0 = &H0009
Const W5100_shar1 = &H000A
Const W5100_shar2 = &H000B
Const W5100_shar3 = &H000C
Const W5100_shar4 = &H000D
Const W5100_shar5 = &H000E
'Source IP Address registers
Const W5100_sipr0 = &H000F
Const W5100_sipr1 = &H0010
Const W5100_sipr2 = &H0011
Const W5100_sipr3 = &H0012
Dim Value As Byte
Dim Adres As Word
Dim Adresl As Byte At Adres Overlay
Dim Adresh As Byte At Adres + 1 Overlay
' 0 = long board
' 1 = sandwichboard
Const Board = 1
'Used Wiz5100 ports and pins
Wiz5100_cs Alias Portb.2
'Chipselect WIZ5100
#if Board
'sandwichboard
Wiz5100_res Alias Portd.3
'reset of WIZ810MJ
'Other used ports and pins
Relais1 Alias Portd.4
Relais2 Alias Portd.5
Relais3 Alias Portd.6
Relais4 Alias Portd.7
#elseif
Wiz5100_res Alias Portc.0 'long board
Relais1 Alias Portc.1
Relais2 Alias Portc.2
Relais3 Alias Portc.3
Relais4 Alias Portc.4
#endif
'Used ports and pins
Config Wiz5100_cs = Output
Config Wiz5100_res = Output
'Configuration of the SPI-bus
Config Spi = Hard , Interrupt = Off , Data Order = Msb , Master = Yes , Polarity = Low , Phase = 0 , Clockrate = 4 , Noss = 0
'Init the spi pins
Spiinit
'Here we declare the used sub routines
Declare Sub Wiz5100_init
Declare Sub Wiz5100_readvalue(byval Reg As Word)
Declare Sub Wiz5100_writevalue(byval Reg As Word , Byval Value As Byte)
Declare Sub Wiz5100_reset

Dim Wiz5100_opcode_read As Byte
Wiz5100_opcode_read = 15
Dim Wiz5100_opcode_write As Byte
Wiz5100_opcode_write = 240
Call Wiz5100_init
'We initialize the Wiz5100
Do
Loop
End
Sub Wiz5100_init
Call Wiz5100_reset
'Hardware reset
'Register reset
Call Wiz5100_writevalue(W5100_mr , &H80)
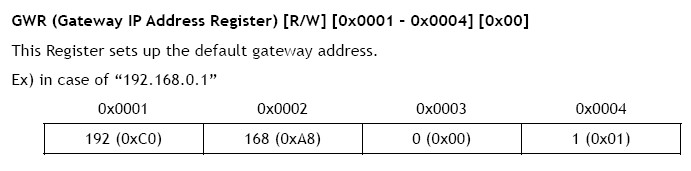
'Set gateway IP address
Call Wiz5100_writevalue(W5100_gar0 , 192)
Call Wiz5100_writevalue(W5100_gar1 , 168)
Call Wiz5100_writevalue(W5100_gar2 , 0)
Call Wiz5100_writevalue(W5100_gar3 , 254)
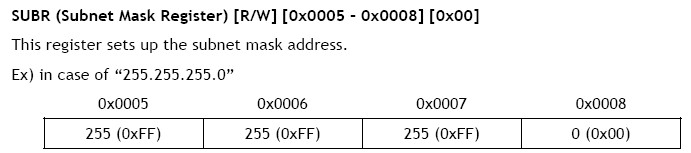
'Set Subnet mask
Call Wiz5100_writevalue(W5100_subr0 , 255)
Call Wiz5100_writevalue(W5100_subr1 , 255)
Call Wiz5100_writevalue(W5100_subr2 , 255)
Call Wiz5100_writevalue(W5100_subr3 , 0)
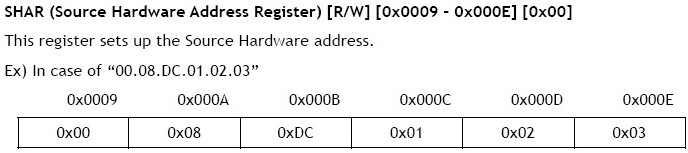
'Set MAC
Call Wiz5100_writevalue(W5100_shar0 , &H00)
Call Wiz5100_writevalue(W5100_shar1 , &H10)
Call Wiz5100_writevalue(W5100_shar2 , &H20)
Call Wiz5100_writevalue(W5100_shar3 , &H30)
Call Wiz5100_writevalue(W5100_shar4 , &H40)
Call Wiz5100_writevalue(W5100_shar5 , &H50)
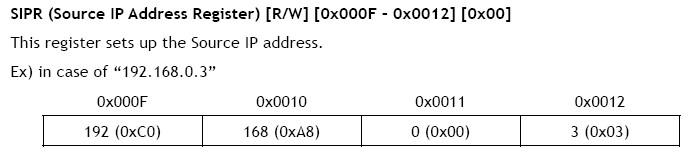
'Set own IP address
Call Wiz5100_writevalue(W5100_sipr0 , 192)
Call Wiz5100_writevalue(W5100_sipr1 , 168)
Call Wiz5100_writevalue(W5100_sipr2 , 0)
Call Wiz5100_writevalue(W5100_sipr3 , 73)
End Sub
Sub Wiz5100_readvalue(reg)
Adres = Reg
Reset Wiz5100_cs
Spiout Wiz5100_opcode_read , 1
Spiout Adresh , 1
Spiout Adresl , 1
Spiin Value , 1
Set Wiz5100_cs
End Sub
Sub Wiz5100_writevalue(reg , Value )
Adres = Reg
Reset Wiz5100_cs
Spiout Wiz5100_opcode_write , 1
Spiout Adresh , 1
Spiout Adresl , 1
Spiout Value , 1
Set Wiz5100_cs
End Sub
Sub Wiz5100_reset
Wiz5100_res = 1
Waitms 10
Wiz5100_res = 0
Waitms 30
'Minimum 20 µs
Wiz5100_res = 1
End Sub
We are using the internal clock
of the Atmega168. With the CONST we are mapping registers to address locations. Later on we will put all constants in a
separate INClude-file.
Start a PING and you will see the
reaction of the WIZ810MJ-module.
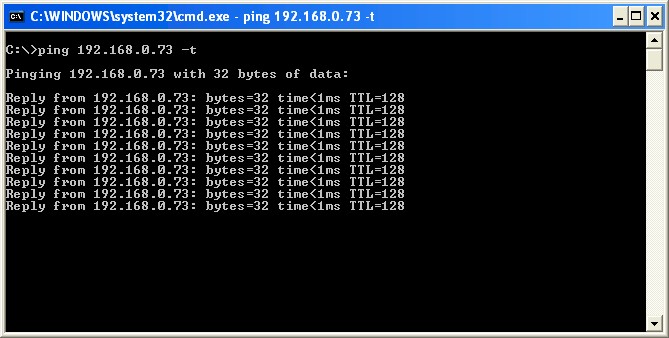
|
Include
file - W5100.INC.... |
Have put all registers in an Include
file.
$include
"W5100.inc"
|
UDP, a few extra lines: |
'now open a UDP-socket
'Assign all 8k memory to socket 0 for RX
Call Wiz5100_writevalue(w5100_rmsr , &B00000011)
'Assign all 8k memory to socket 0 for TX
Call Wiz5100_writevalue(w5100_tmsr , &B00000011)
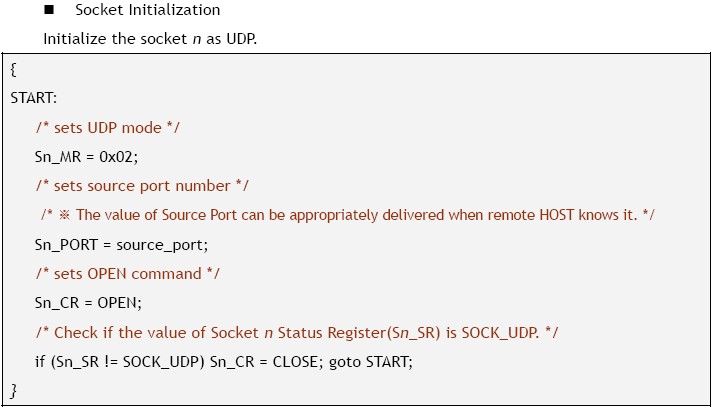
'protocol = UDP
Call Wiz5100_writevalue(w5100_s0_mr , Sn_mr_udp)
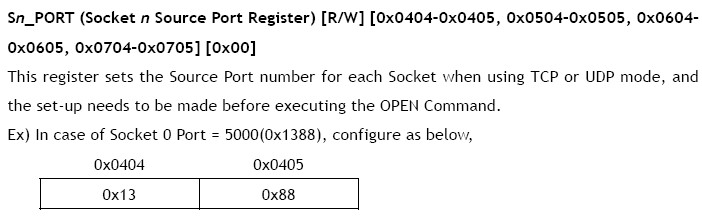
Call Wiz5100_writevalue(w5100_s0_port0 , &H13)
' port 5000
Call Wiz5100_writevalue(w5100_s0_port1 , &H88)
'give the open command
Call Wiz5100_writevalue(w5100_s0_cr , Sn_cr_open)
'wait for socket to come online
Do

Print "Value = " ; Value
Call Wiz5100_readvalue(w5100_s0_sr)
Loop Until Value = Sock_udp
Print "value = " ; Value
Call Wiz5100_readvalue(&H6000)
Print "6000" ; " " ; Chr(value) ; " " ; Value
etc.etc
In a terminal you will get this
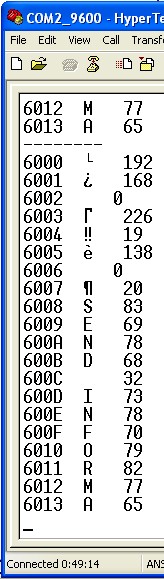
I have used the Ethernet I/O Control
Panel that, with an interval, the UDP string SEND INFORMATION NOW sends.
In the above screen in the buffer of the WIZ810MJ on address &H6000
first the IP-address of the sender 192.168.0.226, followed by the
destination port 5002 (19 = &H13, 138 = &H8A : &H138A =
5002). Followed by the length of the UDP string (20) and then the
UDP-string itself.
The Ethernet I/O Control Panel can be
downloaded at www.drftech.com
UDP working, 2206 bytes used.
Here the program so far, and some extra
info from the datasheet:
'--------------------------------------------------------------
' Atmega168 and WIZ810MJ PING and UDP working
'--------------------------------------------------------------
$regfile = "m168def.dat"
$crystal = 8000000
$hwstack = 64
$swstack = 64
$framesize = 64
$include "w5100.inc"
Dim Value As Byte
Dim Adres As Word
Dim Adresl As Byte At Adres Overlay
Dim Adresh As Byte At Adres + 1 Overlay
' 0 = long board
' 1 = sandwichboard
Const Board = 1
'Used Wiz5100 ports and pins
Wiz5100_cs Alias Portb.2 'Chipselect WIZ5100
#if Board 'sandwichboard
Wiz5100_res Alias Portd.3 'reset of WIZ810MJ
'Other used ports and pins
Relais1 Alias Portd.4
Relais2 Alias Portd.5
Relais3 Alias Portd.6
Relais4 Alias Portd.7
#elseif
Wiz5100_res Alias Portc.0 'long board
Relais1 Alias Portc.1
Relais2 Alias Portc.2
Relais3 Alias Portc.3
Relais4 Alias Portc.4
#endif
'Used ports and pins
Config Wiz5100_cs = Output
Config Wiz5100_res = Output
'Configuration of the SPI-bus
Config Spi = Hard , Interrupt = Off , Data Order = Msb , Master = Yes , Polarity = Low , Phase = 0 , Clockrate = 4 , Noss = 0
'Init the spi pins
Spiinit
'Here we declare the used sub routines
Declare Sub Wiz5100_init
Declare Sub Wiz5100_readvalue(byval Reg As Word)
Declare Sub Wiz5100_writevalue(byval Reg As Word , Byval Value As Byte)
Declare Sub Wiz5100_reset
Dim Wiz5100_opcode_read As Byte
Wiz5100_opcode_read = 15
Dim Wiz5100_opcode_write As Byte
Wiz5100_opcode_write = 240
Call Wiz5100_init
'We initialize the Wiz5100
'now open a UDP-socket
'Assign all 8k memory to socket 0 for RX
Call Wiz5100_writevalue(w5100_rmsr , &B00000011)
'Assign all 8k memory to socket 0 for TX
Call Wiz5100_writevalue(w5100_tmsr , &B00000011)
'protocol = UDP
Call Wiz5100_writevalue(w5100_s0_mr , Sn_mr_udp)
Call Wiz5100_writevalue(w5100_s0_port0 , &H13) ' port 5000
Call Wiz5100_writevalue(w5100_s0_port1 , &H88)
'give the open command
Call Wiz5100_writevalue(w5100_s0_cr , Sn_cr_open)
'wait for socket to come online
Do
'test
Print "Value = " ; Value
Call Wiz5100_readvalue(w5100_s0_sr)
Loop Until Value = Sock_udp
Print "value = " ; Value
Do
For X = &H6000 To &H601C
Call Wiz5100_readvalue(x)
Print hex(X) ; " " ; Chr(value) ; " " ; Value
Next X
Here you can get the size of the
UDP-packet just received
'Socket 0 RX Received Size
Call Wiz5100_readvalue(w5100_s0_rx_rsr0)
Print "Size received data " ; Value
Call Wiz5100_readvalue(w5100_s0_rx_rsr1)
Print "Size received data " ; Value
Here you can see where the read-pointer
is
'Socket 0 RX Read Pointer
Call Wiz5100_readvalue(w5100_s0_rx_rd0)
Print "Readpointer " ; Value
Call Wiz5100_readvalue(w5100_s0_rx_rd1)
Print "Readpointer " ; Value
Wait 10
Print "--------"
Loop
End
Sub Wiz5100_init
Call Wiz5100_reset 'Hardware reset
'Register reset
Call Wiz5100_writevalue(w5100_mr , &H80)
'Set gateway IP adress
Call Wiz5100_writevalue(w5100_gar0 , 192)
Call Wiz5100_writevalue(w5100_gar1 , 168)
Call Wiz5100_writevalue(w5100_gar2 , 0)
Call Wiz5100_writevalue(w5100_gar3 , 254)
'Set Subnetmask
Call Wiz5100_writevalue(w5100_subr0 , 255)
Call Wiz5100_writevalue(w5100_subr1 , 255)
Call Wiz5100_writevalue(w5100_subr2 , 255)
Call Wiz5100_writevalue(w5100_subr3 , 0)
'Set MAC
Call Wiz5100_writevalue(w5100_shar0 , &H00)
Call Wiz5100_writevalue(w5100_shar1 , &H10)
Call Wiz5100_writevalue(w5100_shar2 , &H20)
Call Wiz5100_writevalue(w5100_shar3 , &H30)
Call Wiz5100_writevalue(w5100_shar4 , &H40)
Call Wiz5100_writevalue(w5100_shar5 , &H50)
'Set own IP adress
Call Wiz5100_writevalue(w5100_sipr0 , 192)
Call Wiz5100_writevalue(w5100_sipr1 , 168)
Call Wiz5100_writevalue(w5100_sipr2 , 0)
Call Wiz5100_writevalue(w5100_sipr3 , 73)
End Sub
Sub Wiz5100_readvalue(reg)
Adres = Reg
Reset Wiz5100_cs
Spiout Wiz5100_opcode_read , 1
Spiout Adresh , 1
Spiout Adresl , 1
Spiin Value , 1
Set Wiz5100_cs
End Sub
Sub Wiz5100_writevalue(reg , Value )
Adres = Reg
Reset Wiz5100_cs
Spiout Wiz5100_opcode_write , 1
Spiout Adresh , 1
Spiout Adresl , 1
Spiout Value , 1
Set Wiz5100_cs
End Sub
Sub Wiz5100_reset
Wiz5100_res = 1
Waitms 10
Wiz5100_res = 0
Waitms 30 'Minimum 20 µs
Wiz5100_res = 1
End Sub
With the tool from Hugh Duff I get 28
characters each time.
|
A small UDP application: |
CC 29 FE E0
Going to do a small experiment.
Get the EDTP Electronics Internet Test
Panel from www.edtp.com
Start you network sniffer (Etherreal,
Wireshark, Iris etc. etc.)
Send a X to a timeserver, here I use
193.67.79.202, a timeserver here in Holland, on port 37.
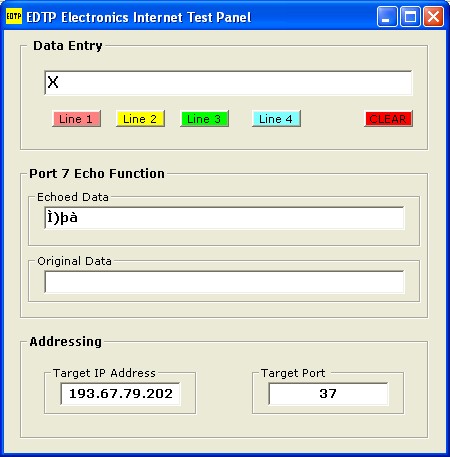
If all goes well you will get a reply,
at the time I started the UDP-transmission I got a four hex-digits: CC
29 FE E0
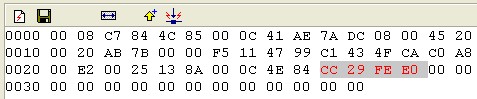
Startup Bascom-AVR, type in the
program, and run it in the simulator.
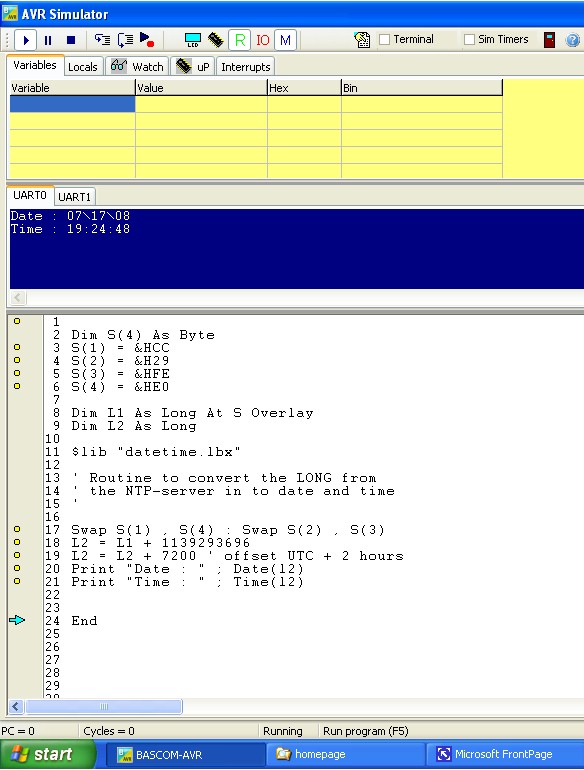
You will get: Date: 07/17/08, Time:
19:24
7200 = 2 hours from GMT.
And about the 1139293696 you can read
about it here:
https://unix.derkeiler.com/Newsgroups/comp.unix.shell/2006-09/msg00253.html
|
Receiving
on Socket 0 and getting an interrupt... |
In the WizNet forum I have read that the
combination SPI and an hardware Interrupt should work. Have spend some
time but can not get the hardware Interrupt working. In the datasheet,
SPI-connection, no hardware interrupt line is used.
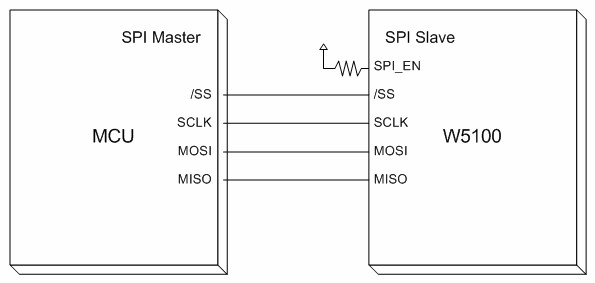
So how to detect there is something
received on Socket 0
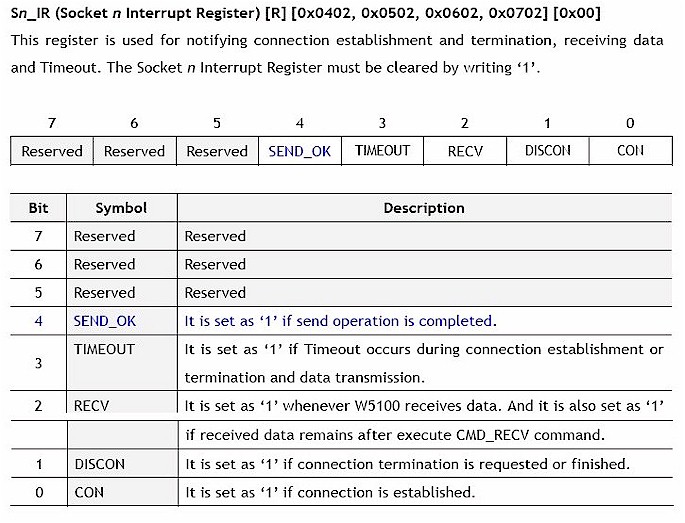
This simple routine:
'only on socket 0
Call Wiz5100_writevalue(w5100_imr , &B0000_0001)
'check on RECV
Do
Call Wiz5100_readvalue(w5100_s0_ir)
Print Bin(value)
If Value.2 = 1 Then
Print "Received something on Socket 0"
Call Wiz5100_writevalue(w5100_s0_ir , &B0000_0100)
End If
Loop
We first mask the interrupt we want to
have. After that we want to check if something is received. When
something is received bit 2 of the W5100_S0_ir is set, and by writing a
'1' to it we clear this bit again.
|
Sending
a X CR/LF to receive the time from a NTP-server.... |
' Sending a UDP-packet
'IP-number
Call Wiz5100_writevalue(w5100_s0_dipr0 , 193) 'IP-number
of NTP-server in Holland
Call Wiz5100_writevalue(w5100_s0_dipr1 , 67)
Call Wiz5100_writevalue(w5100_s0_dipr2 , 79)
Call Wiz5100_writevalue(w5100_s0_dipr3 , 202)
'Destination port
Call Wiz5100_writevalue(w5100_s0_dport0 , 0)
Call Wiz5100_writevalue(w5100_s0_dport1 , 37)
'port 37
'Data
Call Wiz5100_writevalue(&H4000 , 88)
' X
Call Wiz5100_writevalue(&H4001 , &H0D)
'carriage return
Call Wiz5100_writevalue(&H4002 , &H0A)
'line feed
'set size
Call Wiz5100_writevalue(w5100_s0_tx_wr0 , 0)
Call Wiz5100_writevalue(w5100_s0_tx_wr1 , 3)
' size of the packet
' send
Wait 1
'
Call Wiz5100_writevalue(w5100_s0_cr , Sn_cr_send)
Do
Call Wiz5100_readvalue(w5100_s0_cr)
Loop Until Value = 0
Print "X CR/LF should have been send"
Print
'let's have a look if interrupt is working while using SPI
'only on socket 0
Call Wiz5100_writevalue(w5100_imr , &B0000_0001)
'check on RECV
Do
Call Wiz5100_readvalue(w5100_s0_ir)
'Print Bin(value)
If Value.2 = 1 Then
Print "Received something on Socket 0"
Call Wiz5100_writevalue(w5100_s0_ir , &B100)
Exit Do
End If
Loop

Do
For X = &H6000 To &H601C
Call Wiz5100_readvalue(x)
Print Hex(x) ; " " ; Chr(value) ; " " ; Value
Next X
'Socket 0 RX Received Size
Call Wiz5100_readvalue(w5100_s0_rx_rsr0)
Print "Size received data " ; Value
Call Wiz5100_readvalue(w5100_s0_rx_rsr1)
Print "Size received data " ; Value
'Socket 0 RX Read Pointer
Call Wiz5100_readvalue(w5100_s0_rx_rd0)
Print "Readpointer " ; Value
Call Wiz5100_readvalue(w5100_s0_rx_rd1)
Print "Readpointer " ; Value
Wait 10
Print "--------"
Loop
End
16 bytes received back from the
time-server.
CC 33 80 D0
|
Receiving
UDP... |
Dim Temp As Word
Dim Size As Word
Dim Sizel As Byte At Size Overlay
Dim Sizeh As Byte At Size + 1 Overlay
Dim Count As Byte
Dim Clock(4) As Byte
Dim L1 As Long At Clock Overlay
Dim L2 As Long
$lib "datetime.lbx"
Config Date = Dmy , Separator = /
Const Gs0_tx_base = &H4000
Const Gs1_tx_base = &H4800
Const Gs2_tx_base = &H5000
Const Gs3_tx_base = &H5800
Const Gs0_rx_base = &H6000
Const Gs1_rx_base = &H6800
Const Gs2_rx_base = &H7000
Const Gs3_rx_base = &H7800
Const Gsn_rx_mask = &H07FF
Const Gsn_tx_mask = &H07FF
'Socket 0 RX Received Size
Call Wiz5100_readvalue(w5100_s0_rx_rsr0)
Sizeh = Value
Call Wiz5100_readvalue(w5100_s0_rx_rsr1)
Sizel = Value
Print "Received bytes " ; Size
Decr Size
Count = 1
Temp = Gs0_rx_base + Size
For X = Gs0_rx_base To Temp
Call Wiz5100_readvalue(x)
Print Hex(x) ; " " ; Hex(value) ; " " ; Value;
If X > &H6007 Then
Print " <<"
Clock(count) = Value
Incr Count
Else
Print
End If
Next X
Print
Swap Clock(1) , Clock(4)
Swap Clock(3) , Clock(2)
L2 = L1 + 1139293696
L2 = L2 + 7200
Print "Date: " ; Date(l2)
Print "Time: " ; Time(l2)
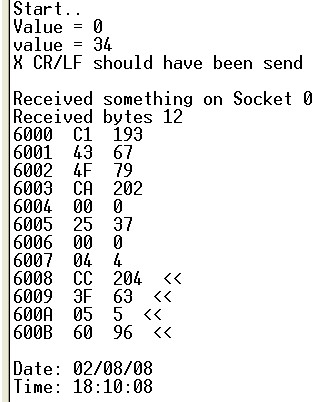
Here the code
so far. In the meantime I switched to the long board,
you can change settings in the code.
' 0 = long board
' 1 = sandwichboard
Const Board = 0
|
Read/Write
pointer and freesize |
'
Print "TX Write pointer"
Call Wiz5100_readvalue(w5100_s0_tx_wr0)
Print "W5100_s0_tx_wr0 = " ; Hex(value)
Call Wiz5100_readvalue(w5100_s0_tx_wr1)
Print "W5100_s0_tx_wr1 = " ; Hex(value)
'
Print "TX Read pointer"
Call Wiz5100_readvalue(w5100_s0_tx_rd0)
Print "W5100_s0_tx_rd0 = " ; Hex(value)
Call Wiz5100_readvalue(w5100_s0_tx_rd1)
Print "W5100_s0_tx_rd1 = " ; Hex(value)
'
Print "Freesize"
Call Wiz5100_readvalue(w5100_s0_tx_fsr0)
Print "W5100_s0_tx_fsr0 = " ; Hex(value)
Call Wiz5100_readvalue(w5100_s0_tx_fsr1)
Print "W5100_s0_tx_fsr1 = " ; Hex(value)
'
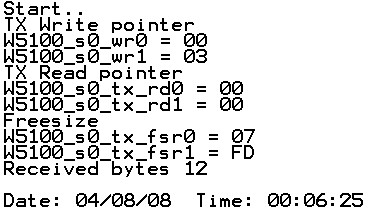
|
Socket
registers... |
Example: Every socket 2
Kbyte.
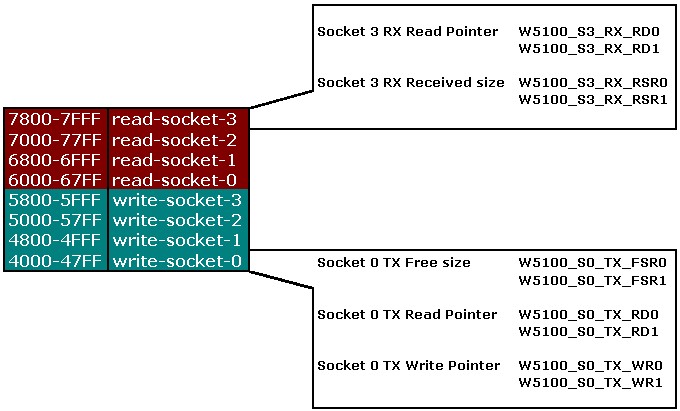
Several registers for
the sockets
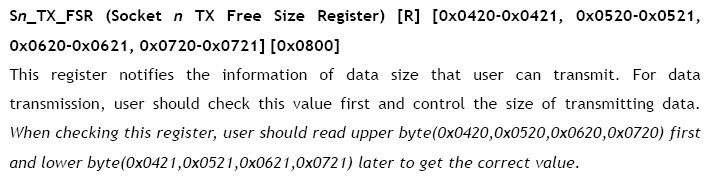
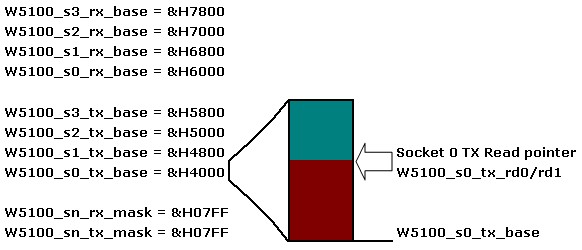
TX read pointer sliding
through the memory.
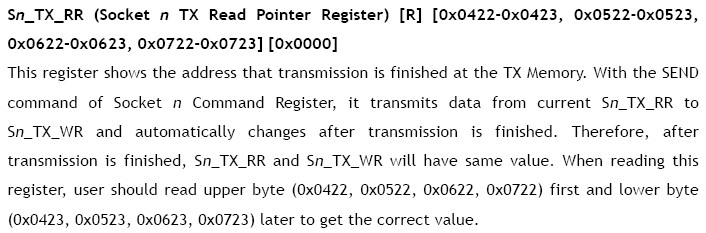
I am using datasheet
W5100_Datasheet v1.1.5.PDF In it two different names for registers
&H422/423, &H522/523, &H622/623 and &H722/723.
Sn_TX_RD0 and Sn_TX_RD1
or Sn_TX_RR0 and Sn_TX_RR1
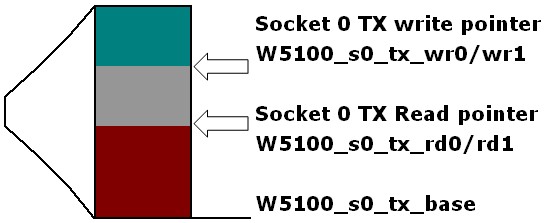
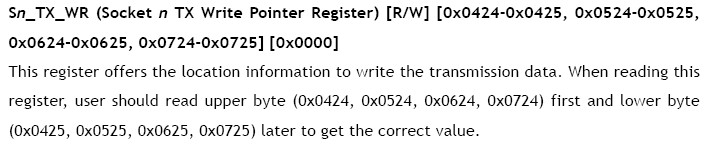
The complete code to get
a NTP-client working
'------------------------------------------------------------------
' Atmega168 and WIZ810MJ All you need to get a PING and NTP working
'------------------------------------------------------------------
$regfile = "m168def.dat"
$crystal = 8000000
$baud = 9600
$hwstack = 64
$swstack = 64
$framesize = 64
$loadersize = 2048
$include "w5100.inc"
Dim Value As Byte
Dim Adres As Word
Dim Adresl As Byte At Adres Overlay
Dim Adresh As Byte At Adres + 1 Overlay
Dim X As Word
Dim Temp As Word
Dim Size As Word
Dim Sizel As Byte At Size Overlay
Dim Sizeh As Byte At Size + 1 Overlay
Dim Count As Byte
Dim Clock(4) As Byte
Dim L1 As Long At Clock Overlay
Dim L2 As Long
$lib "datetime.lbx"
Config Date = Dmy , Separator = /
Const Gs0_rx_base = &H6000
' 0 = long board
' 1 = sandwichboard
Const Board = 1
'Used Wiz5100 ports and pins
Wiz5100_cs Alias Portb.2 'Chipselect WIZ810MJ
Print "Start.."
#if Board 'sandwichboard
Wiz5100_res Alias Portd.3 'reset of WIZ810MJ
'Other used ports and pins
Relais1 Alias Portd.4
Relais2 Alias Portd.5
Relais3 Alias Portd.6
Relais4 Alias Portd.7
#elseif
Wiz5100_res Alias Portc.0 'long board
Relais1 Alias Portc.1
Relais2 Alias Portc.2
Relais3 Alias Portc.3
Relais4 Alias Portc.4
#endif
'Used ports and pins
Config Wiz5100_cs = Output
Config Wiz5100_res = Output
Config Lcdpin = Pin , Db4 = Portc.2 , Db5 = Portc.3 , Db6 = Portc.4 , Db7 = Portc.5 , E = Portc.1 , Rs = Portc.0
Config Lcd = 16 * 2
'Configuration of the SPI-bus
Config Spi = Hard , Interrupt = Off , Data Order = Msb , Master = Yes , Polarity = Low , Phase = 0 , Clockrate = 4 , Noss = 0
'Init the spi pins
Spiinit
'Here we declare the used sub routines
Declare Sub Wiz5100_init
Declare Sub Wiz5100_readvalue(byval Reg As Word)
Declare Sub Wiz5100_writevalue(byval Reg As Word , Byval Value As Byte)
Declare Sub Wiz5100_reset
Dim Wiz5100_opcode_read As Byte
Wiz5100_opcode_read = 15
Dim Wiz5100_opcode_write As Byte
Wiz5100_opcode_write = 240
Call Wiz5100_init 'We initialize the Wiz810MJ
'now open a UDP-socket
'Assign all 2k memory to every socket for RX
Call Wiz5100_writevalue(w5100_rmsr , &B01010101) '
'Assign all 2k memory to every socket for TX
Call Wiz5100_writevalue(w5100_tmsr , &B01010101)
'protocol = UDP
Call Wiz5100_writevalue(w5100_s0_mr , Sn_mr_udp)
Call Wiz5100_writevalue(w5100_s0_port0 , &H13) ' port 5000
Call Wiz5100_writevalue(w5100_s0_port1 , &H88)
'give the open command
Call Wiz5100_writevalue(w5100_s0_cr , Sn_cr_open)
'wait for socket to come online
Do
Call Wiz5100_readvalue(w5100_s0_sr)
Loop Until Value = Sock_udp
' Sending a UDP-packet
'IP-number
Call Wiz5100_writevalue(w5100_s0_dipr0 , 193)
Call Wiz5100_writevalue(w5100_s0_dipr1 , 67)
Call Wiz5100_writevalue(w5100_s0_dipr2 , 79)
Call Wiz5100_writevalue(w5100_s0_dipr3 , 202)
'Destination port
Call Wiz5100_writevalue(w5100_s0_dport0 , 0)
Call Wiz5100_writevalue(w5100_s0_dport1 , 37)
'Data
Call Wiz5100_writevalue(&H4000 , 88) ' X
Call Wiz5100_writevalue(&H4001 , &H0D)
Call Wiz5100_writevalue(&H4002 , &H0A)
'set size
Call Wiz5100_writevalue(w5100_s0_tx_wr0 , 0)
Call Wiz5100_writevalue(w5100_s0_tx_wr1 , 3)
' send
Wait 1
'
Call Wiz5100_writevalue(w5100_s0_cr , Sn_cr_send)
Do
Call Wiz5100_readvalue(w5100_s0_cr)
Loop Until Value = 0
Print "X CR/LF send"
'let's have a look if interrupt is working while using SPI
'only on socket 0
Call Wiz5100_writevalue(w5100_imr , &B0000_0001)
'check on RECV
Do
Call Wiz5100_readvalue(w5100_s0_ir)
Print Bin(value)
If Value.2 = 1 Then
Print "Rec. on Sck 0"
Call Wiz5100_writevalue(w5100_s0_ir , &B100)
Exit Do
End If
Loop
'Socket 0 RX Received Size
Call Wiz5100_readvalue(w5100_s0_rx_rsr0)
Sizeh = Value
Call Wiz5100_readvalue(w5100_s0_rx_rsr1)
Sizel = Value
Print "Received bytes " ; Size
Decr Size
Count = 1
Temp = Gs0_rx_base + Size
For X = Gs0_rx_base To Temp
Call Wiz5100_readvalue(x)
Print Hex(x) ; " " ; Hex(value) ; " " ; Value;
If X > &H6007 Then
Print " <<"
Clock(count) = Value
Incr Count
Else
Print
End If
Next X
Print
Swap Clock(1) , Clock(4)
Swap Clock(3) , Clock(2)
L2 = L1 + 1139293696
L2 = L2 + 7200
Cls
Home
Lcd "Date: " ; Date(l2)
Lowerline
Lcd "Time: " ; Time(l2)
End
Sub Wiz5100_init
Call Wiz5100_reset 'Hardware reset
'Register reset
Call Wiz5100_writevalue(w5100_mr , &H80)
'Set gateway IP adress
Call Wiz5100_writevalue(w5100_gar0 , 192)
Call Wiz5100_writevalue(w5100_gar1 , 168)
Call Wiz5100_writevalue(w5100_gar2 , 0)
Call Wiz5100_writevalue(w5100_gar3 , 254)
'Set Subnetmask
Call Wiz5100_writevalue(w5100_subr0 , 255)
Call Wiz5100_writevalue(w5100_subr1 , 255)
Call Wiz5100_writevalue(w5100_subr2 , 255)
Call Wiz5100_writevalue(w5100_subr3 , 0)
'Set MAC
Call Wiz5100_writevalue(w5100_shar0 , &H00)
Call Wiz5100_writevalue(w5100_shar1 , &H10)
Call Wiz5100_writevalue(w5100_shar2 , &H20)
Call Wiz5100_writevalue(w5100_shar3 , &H30)
Call Wiz5100_writevalue(w5100_shar4 , &H40)
Call Wiz5100_writevalue(w5100_shar5 , &H50)
'Set own IP adress
Call Wiz5100_writevalue(w5100_sipr0 , 192)
Call Wiz5100_writevalue(w5100_sipr1 , 168)
Call Wiz5100_writevalue(w5100_sipr2 , 0)
Call Wiz5100_writevalue(w5100_sipr3 , 50)
End Sub
Sub Wiz5100_readvalue(reg)
Adres = Reg
Reset Wiz5100_cs
Spiout Wiz5100_opcode_read , 1
Spiout Adresh , 1
Spiout Adresl , 1
Spiin Value , 1
Set Wiz5100_cs
End Sub
Sub Wiz5100_writevalue(reg , Value )
Adres = Reg
Reset Wiz5100_cs
Spiout Wiz5100_opcode_write , 1
Spiout Adresh , 1
Spiout Adresl , 1
Spiout Value , 1
Set Wiz5100_cs
End Sub
Sub Wiz5100_reset
Wiz5100_res = 1
Waitms 10
Wiz5100_res = 0
Waitms 30 'Minimum 20 µs
Wiz5100_res = 1
End Sub
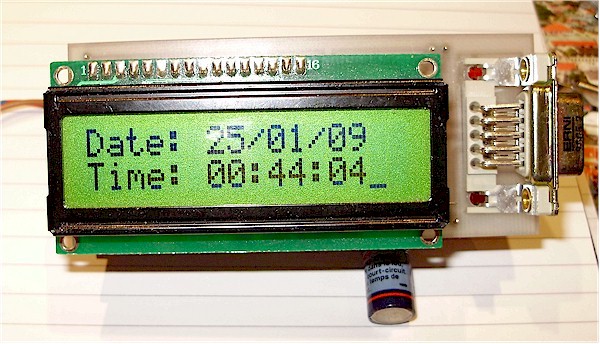
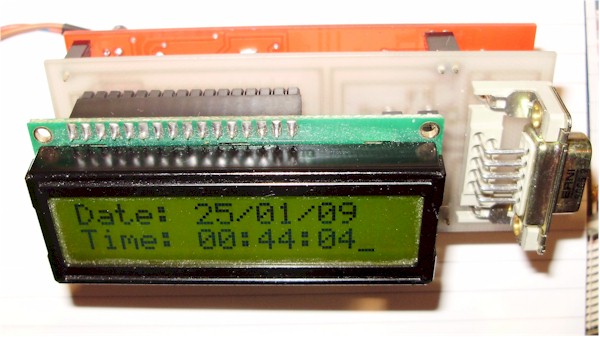
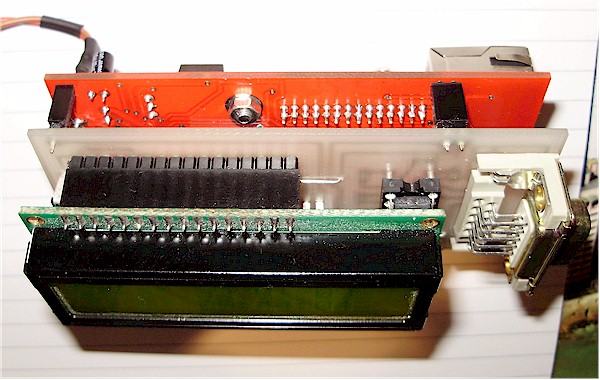
Prototype of a LCD-board
for the Red Bokito.
With RS232, optocouplers, 7805 voltageregulator.
With the NTP-example.
|
Receiving
UDP-data... |
Dim Wtempb As Word
Dim Wtempe As Word
Dim Size As Word
Dim Sizel As Byte At Size Overlay
Dim Sizeh As Byte At Size + 1 Overlay
Dim Pointer As Word
Dim Pointerl As Byte At Pointer Overlay
Dim Pointerh As Byte At Pointer + 1 Overlay
Dim Bpointer As Word
Dim Epointer As Word
'check on RECV
Do
Call Wiz5100_readvalue(w5100_s0_ir)
If Value.2 = 1 Then
Print "Received something on Socket 0"
Print
'read the Read Pointer
'Print "Read RX_RD0/1"
Call Wiz5100_readvalue(w5100_s0_rx_rd0)
'Print
"W5100_s0_rx_rd0 " ; Hex(value)
Pointerh = Value
Call Wiz5100_readvalue(w5100_s0_rx_rd1)
'Print
"W5100_s0_rx_rd1 " ; Hex(value)
Pointerl = Value
'Print "Read Pointer = " ;
Hex(pointer)
Bpointer = Pointer
'Socket 0 RX Received Size
Call Wiz5100_readvalue(w5100_s0_rx_rsr0)
Sizeh = Value
Call Wiz5100_readvalue(w5100_s0_rx_rsr1)
Sizel = Value
Pointer = Pointer + Size
Epointer = Pointer
Print "Begin is = " ; Bpointer
Print "End is = " ; Epointer
Print "Size is = " ; Size
Wtempb = W5100_s0_rx_base + Bpointer
Wtempe = Wtempb + Size
'we still have to take care of the MASK of &H07FF (later)
Print "Begin WIZ810MJ RX-memory = " ;
Hex(wtempb)
Print "End WIZ810MJ RX-memory = " ;
Hex(wtempe)
Print
'skip 8 byte header
Wtempb = Wtempb + 8
Decr Wtempe
Print "Received
UDP-string"
Print
For X = Wtempb To Wtempe
Call Wiz5100_readvalue(x)
Print Chr(value);
Next X
Print
Print
'update pointer
Call Wiz5100_writevalue(w5100_s0_rx_rd0 , Pointerh)
Call Wiz5100_writevalue(w5100_s0_rx_rd1 , Pointerl)
Call Wiz5100_writevalue(w5100_s0_cr ,
Sn_cr_recv)
'reset interrupt
Call Wiz5100_writevalue(w5100_s0_ir , &B100)
End If
Loop
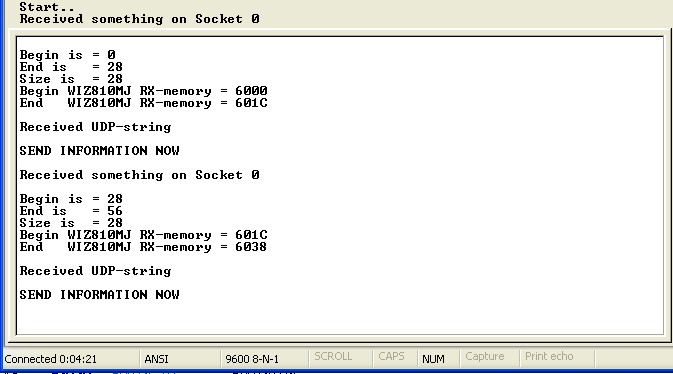
The big
picture... We have made a loop and are polling the interrupt-register
w5100_s0_ir. If bit 2 is set, something has been received. We
store the RX-read pointer in pointer, the size of the received
UDP-packet in size. We calculate the start in the RX-memory (adding
the W5100_s0_rx_base), we calculate the end of the UDP-packet.
Then we skip the 8-byte header and read the contents of the UDP-packet.
After that we update the RX-read pointer and do a reset of the interrupt.
That's all. We still have to take care of the size op the circular
RX-memory (&H0800). Have to optimize the code and make it available
for all sockets. Not that difficult, all is handled within the routine,
the old RX-memory pointer, the size of the UDP-packet and updating the
new RX-memory pointer.
In the example I have started the Ethernet I/O panel from Hugh Duff with
an interval of 10 seconds, IP-number 192.168.0.50 and port 5000. This
tool sends a string "SEND INFORMATION NOW" every 10 seconds.
Here
the code for this example |
Checking
socket size |
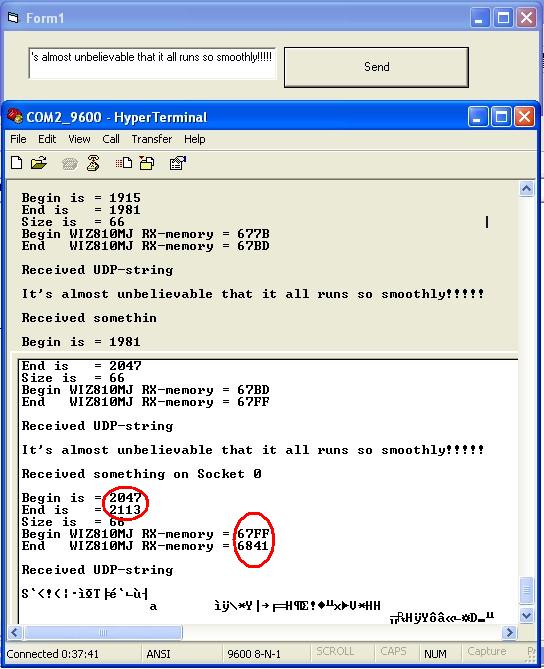
If you go over the boundaries
of your socket (RX-memory) you get the information of the next socket. In
this case, we work in the socket 0 memory and go over the top into
socket 1 memory. So we have to check that we remain in our own socket 0
memory. On the top of the picture my own test program, in Visual Basic
6.0. Just a Winsock-component, a command button and a textbox.
|
UDP
in a circular buffer.... |
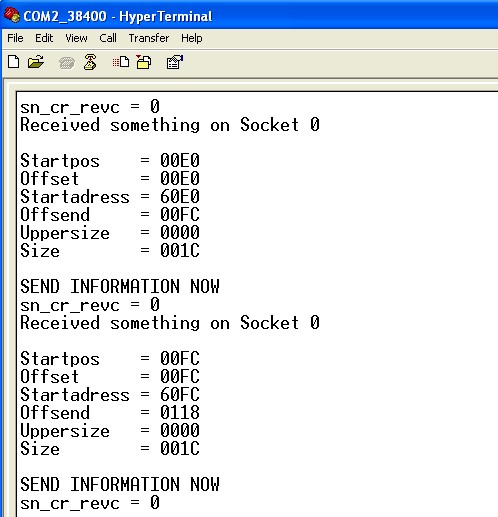
In this picture you can see the receive
pointer at work. It is only a forward step counter so you will go beyond
the boundary of the 2 Kbytes of the Socket 0 receive buffer. Have
started the Ethernet I/O tool from Hugh, and it is sending a UDP message
every 5 seconds. With Startpos, Offset, Startadress, Offsend, Uppersize
and Size you can see exactly what is happening. Especially the Uppersize
is important. Have put the baud rate in the code and the terminal on
38400 baud to keep up.
If Offsend > &H800 Then
Print "Offsend > &H800"
'here
we go over the top
Uppersize = &H800 - Offset
Print "Uppersize nu " ; Hex(uppersize)
Temp = 0
For X = 1 To Uppersize
'skip header 8 byte
Incr Temp
If Temp > 8 Then
Call Wiz5100_readvalue(bpointer)
Print Chr(value);
End If
Incr Bpointer
Next X
Bpointer = &H6000
Incr Uppersize
For X = Uppersize To Size
Incr Temp
If Temp > 8 Then
Call Wiz5100_readvalue(bpointer)
Print Chr(value);
End If
Incr Bpointer
Next X
Else
'here we don't have to go over the top
Temp = 0
For X = 1 To Size
'skip header 8 byte
Incr Temp
If Temp > 8 Then
Call Wiz5100_readvalue(bpointer)
Print Chr(value);
End If
Incr Bpointer
Next X
End If
Print
Here
the code up to this point.
This piece of code
has been written by Thomas Heldt
and you will also see it back in the web server code.
|
Next
step, a web server... |
The next piece of code is a real web server, driving the four relays of the
relay board. It is based on the
small board with the relay board. Baud rate switched back to 9600 baud.
Less than 1000 lines
to get a full web server working
(including a
configuration-screen through the RS232 port)
For those who haven't got the full
Bascom-compiler, I included the HEX-file. It should be used to program
the flash of the Atmega168. The Bascom-AVR can be bought at www.mcselec.com
wiz_webserver.txt
and w5100.inc
wiz_webserver.hex
How about IP-number, net mask, gateway, logon-screen,
names on the buttons etc. etc.
All can be configured in a configuration screen. You get this when you
connect a RS232-cable to your Small board, start HyperTerminal at 9600
baud and press a key.
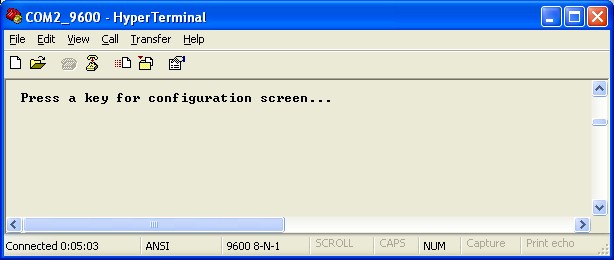
A configuration menu will start and you can enter your
settings.
Because the settings are stored in EEPROM you should put Fuse bit High G on 0.
When erasing flash, to add your own routines, the contents of the EEPROM
will not be erased.
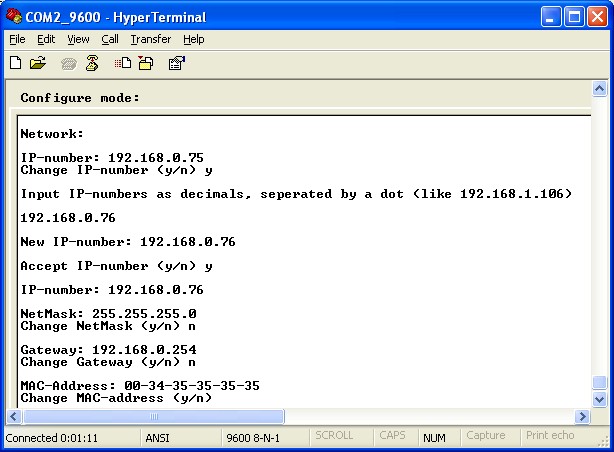
The configuration screen
|
Client
application, a mailer.... |
Perhaps you already know, with Telnet
it is possible to send a mail.
Start/run/cmd (on a
Windows PC)
Telnet 213.51.130.46 25
(213.51.130.46 is the mail server of my
ISP and port 25 is for sending mail).
You will get this
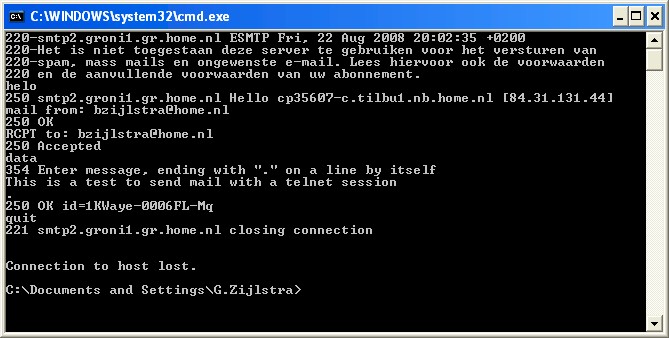
Wouldn't it be nice to have this done
by the Wiz810mj-board? A
switch is turned, you get a mail. A alarm condition, you get a mail.
Threshold to low or to high, mail. We have to make a client application.
This is the flow.
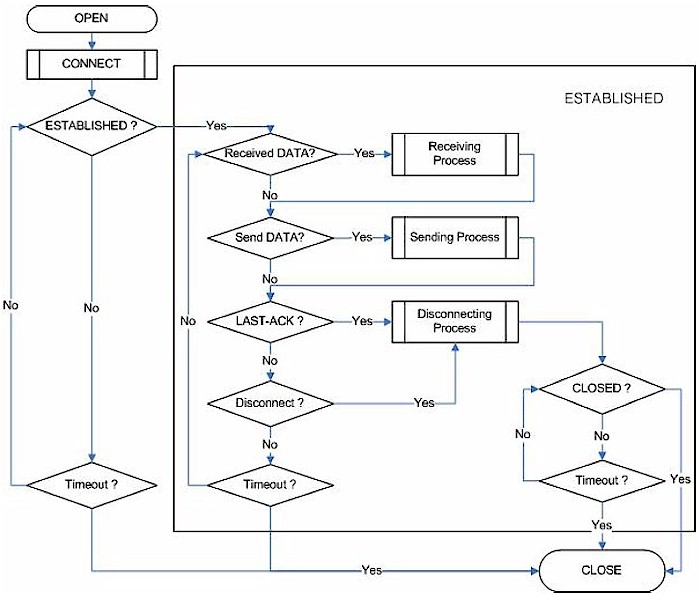
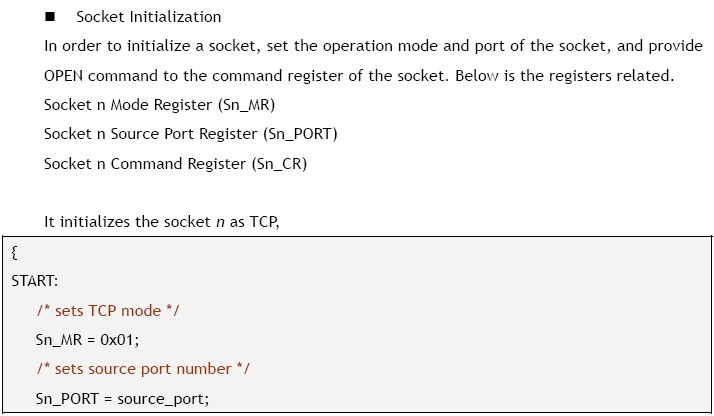
Call Wiz5100_writevalue(w5100_s0_mr , Sn_mr_tcp)
Call Wiz5100_writevalue(w5100_s0_port0 , &H13)
'port 5000
Call Wiz5100_writevalue(w5100_s0_port1 , &H88)

Call Wiz5100_writevalue(w5100_s0_dipr0 , 213) 'IP-number of
mail-server
Call Wiz5100_writevalue(w5100_s0_dipr1 , 51)
Call Wiz5100_writevalue(w5100_s0_dipr2 , 130)
Call Wiz5100_writevalue(w5100_s0_dipr3 , 46)
Call Wiz5100_writevalue(w5100_s0_dport0 , 00)
'port 25
Call Wiz5100_writevalue(w5100_s0_dport1 , 25)
'give the open command
Call Wiz5100_writevalue(w5100_s0_cr , Sn_cr_open)
'wait time to get ARP-request finished
Wait 2
Do
Call Wiz5100_readvalue(w5100_s0_sr)
'sock init?
Loop Until Value = Sock_init
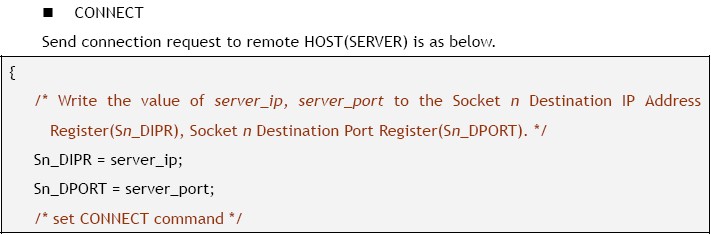
'give the connect command
Call Wiz5100_writevalue(w5100_s0_cr , Sn_cr_connect)
'wait time to get ARP-request finished
Wait 2
Do
Call Wiz5100_readvalue(w5100_s0_sr)
'Sock Established
Loop Until Value = Sock_established
(And now I understand some questions
in the WizNet-forum. What bit should be checked to be sure the
ARP-request is finished successful - now I included twice 2 seconds wait).
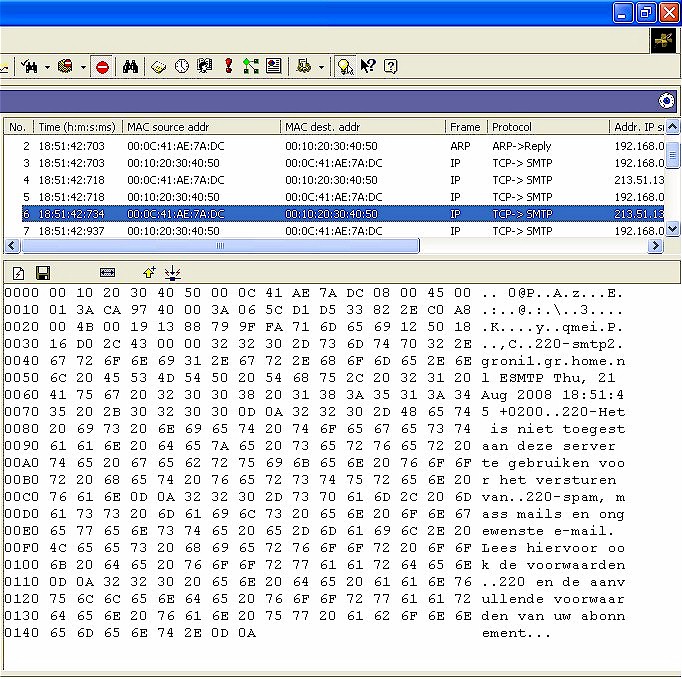
This is the answer from
my ISP mail server. Groningen, Holland.
Translated: It is not permitted to use this server to sent SPAM,
Mass-mail or unwanted mail.
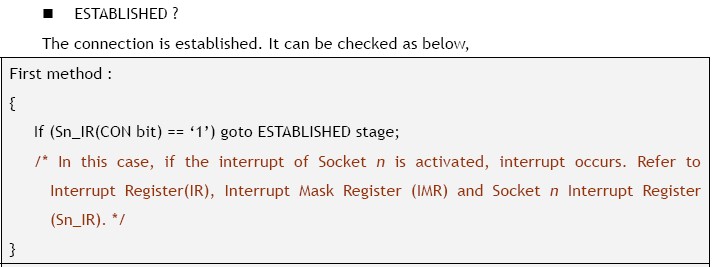


'Set Discon Flag
Call Wiz5100_writevalue(w5100_s0_cr , &H8)
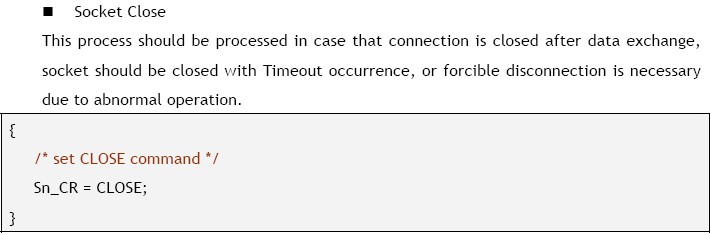
And here the result, a mail from the
Red Bokito in my own mailbox.
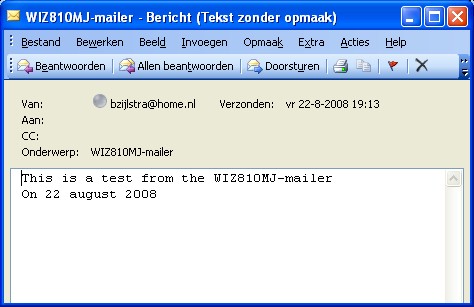
Here
the code for this mailer.
You have to adapt it for your own mail server.
If you want to be absolutely
sure your mail is send, you have to check the status messages you get
from your mail server.
The status messages.
And here the program.
Haven't optimized the code yet. You have to adapt it for your own mail
server.
wiz810mj_mailer2.txt
Watch the details From,
To, CC, BCC
|
and a
mail-retriever.... |
Changing PORT and a few
commands lets us retrieve the mail.
Call Wiz5100_writevalue(w5100_s0_dipr0 , 213)
'IP-number of mail-server
Call Wiz5100_writevalue(w5100_s0_dipr1 , 51)
Call Wiz5100_writevalue(w5100_s0_dipr2 , 146)
Call Wiz5100_writevalue(w5100_s0_dipr3 , 39)
Call Wiz5100_writevalue(w5100_s0_dport0 , 00)
'port 110
Call Wiz5100_writevalue(w5100_s0_dport1 , 110)
Do
Call Wiz5100_readvalue(w5100_s0_sr)
'Sock Established
Loop Until Value = Sock_established
Call Wiz5100_waitfor( "+OK")
'Here we start the conversation
Buffer = "USER bzijlstra@home.nl" + Chr(13) + Chr(10)
Call Wiz5100_sendit
Call Wiz5100_waitfor( "+OK")
Buffer = "PASS xxxxxx" + Chr(13) + Chr(10)
Call Wiz5100_sendit
Call Wiz5100_waitfor( "+OK")
Buffer = "list" + Chr(13) + Chr(10)
Call Wiz5100_sendit
Call Wiz5100_waitfor( "???")
Bcount = Split(reaction , Ar(1) , " ")
Print
Print "You have got " ; Ar(2) ; " messages"
Quit:
Buffer = "quit" + Chr(13) + Chr(10)
Call Wiz5100_sendit
'Set Discon Flag
Call Wiz5100_writevalue(w5100_s0_cr , &H8)
End
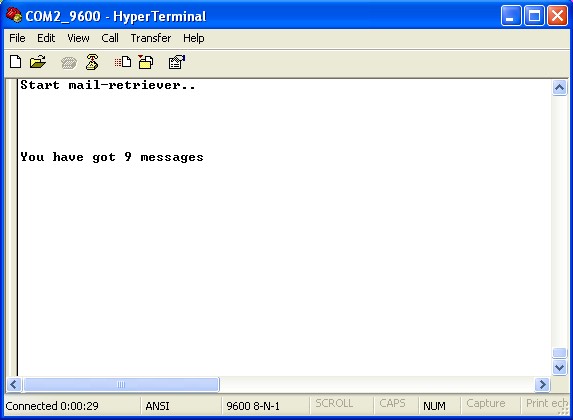
The
code for the mail-retriever
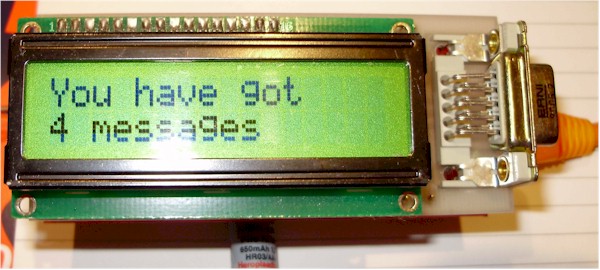
On the LCD prototype
board.
|
About
authentication and passwords.... |
Have noticed Internet Explorer 6.0 and
Internet Explorer 7.0 are both acting different on a authentication of
the webpage. Searching on the Internet I found this
Authentication
differences between IE6 and IE7 - TedMa
|
13-Jun-08
12:56:01
|
We serve secure web sites using Windows 2003 Server SP2 asd IIS 6 in Digest authentication mode using
Network Solutions wildcard certificates. These sites provide information from a number of applications and
servers within frames of the main site.
With IE6 (Automatic login with current username and password), the user could launch any of these applications
without further entering authentication information.
With IE7, the authentication window pops up for every transition, including launching an application within a frame,
then returning to the main page.
I believe this is a programming error in IE7. In IE6, it appears that the browser would check to see if the
Automatic login and password would work, and if they would, proceed to launch the page or frame.
In IE7, it appears that when encountering a new page or frame, the browser pops up the authentication window
without checking to see if the authentication would succeed. This requires the user to constantly be challenged
by the authentication window, rendering the site unusable to IE7 users.
As its unlikely that Microsoft will make any changes so that "Automatic login with current username and password"
will actually work, can anyone provide a work-around for this issue?
|
What about a password on the buttons to
avoid any problems?
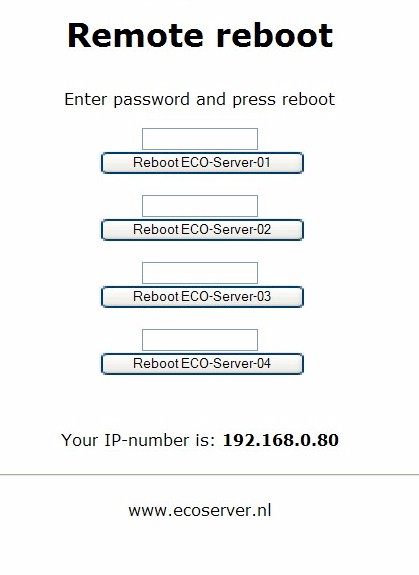
Here a snippet of the code
'Look for variable and replace them, here we can insert new own variables if we want
If Buffer = "%MRELAIS1%" Then
Call Part1
Mid(buffer , X1 ) = "1"
Bfr = System1
Buffer = Buffer + Bfr + Chr(34) + "></form>"
End If
Sub Part1
If Psw = &HFF Then
Buffer = Buffer + "Enter password and press reboot"
End If
Buffer = "<br><form action=" + Chr(34) + "/" + Chr(34) + " method=" + Chr(34) + "POST" + Chr(34) + ">"
If Psw = &HFF Then
Buffer = Buffer + "<input name=" + Chr(34) + "%%%%%%" + Chr(34) + " size=" + Chr(34) + "15" + Chr(34)
Buffer = Buffer + " maxlength=" + Chr(34) + "15" + Chr(34)
Buffer = Buffer + " style=" + Chr(34) + "border-style: solid" + Chr(34)
Buffer = Buffer + " type=" + Chr(34) + "password" + Chr(34) + "><br> "
End If
Buffer = Buffer + "<input type=" + Chr(34) + "hidden" + Chr(34) + " name=" + Chr(34)
Buffer = Buffer + "MRELAIS#"
Buffer = Buffer + Chr(34) + " value=" + Chr(34) + "0" + Chr(34) + ">"
Buffer = Buffer + "<input type=" + Chr(34) + "submit" + Chr(34) + " value=" + Chr(34) + "Reboot "
X1 = Instr(buffer , "#")
X2 = Instr(buffer , "%%%%%%")
End Sub
When I get back from this subroutine,
buffer filled with HTML-code, in X1 the position of the character
#, and in X2 the position of the %%%%%%. # will be replaced by the relays-number,
and %%%%%% wil be replaced by the name for the input text field. The
next step will be replacing the # by the number of relays and %%%%%% by
the systemname fetched from the EEPROM (System1). Is the "Use
password on buttons flag" (PSW) then the MRELAIS will be replaced
by "MPSW01".
If Buffer =
"%MRELAIS1%" Then
Call
Part1
Mid(buffer , X1 ) = "1"
If Psw = &HFF Then
Mid(buffer , X2 ,
6) = "MPSW01"
End If
Bfr = System1
Buffer = Buffer + Bfr + Chr(34) +
"></form>"
End If
Here you can find the passwords.
Perhaps later on I will have them filled in the Configurationscreen and
store them in EEPROM.
If Psw = &HFF
Then
If Instr(buffer , "POST /") = 1 Then
'Toggle RELAIS1
If Instr(buffer
, "MPSW01=test01")
> 0 Then
Call Mrelais1
End If
'Toggle
RELAIS2
If Instr(buffer
, "MPSW02=test02")
> 0 Then
Call Mrelais2
End If
'Toggle
RELAIS3
If Instr(buffer
, "MPSW03=test03")
> 0 Then
Call Mrelais3
End If
'Toggle
RELAIS4
If Instr(buffer ,
"MPSW04=test04")
> 0 Then
Call Mrelais4
End If
End If
|
and
while we are busy, include some temperature sensors... |
We will be using the LM75 I2c
temperature-sensor. I have found this piece of software on a Dutch
Bascom-forum.
Configure the I2c-lines to be used:
'I2c
Config Sda = Portb.7
Config Scl = Portb.6
Declare the subroutines
to be used
'LM75
Declare Sub Dec_in_2er
Declare Sub 2er_in_dec
Declare Sub Read_temp
Declare Sub Resetpointer
Declare Sub Check
Add two new
HTML-instructions
%TEMP1% and %TEMP2%
I have added a few
remarks in the HTML-code
<!--T1--> and
<!--T2-->
With these remarks in
combination with a external program HTML-GET it is possible to retrieve
the temperature of several boards in a single program. Giving the board
some added value.
If Buffer = "%TEMP1%" Then
Lm75read = &H91
Lm75write = &H90
Call Read_temp
Buffer = "<br>Temperature 1 <b><!--T1-->" + Chr(vz) + Str(temp_vk) + "." + Chr(temp_nk) + " C" '
End If
If Buffer = "%TEMP2%" Then
Lm75read = &H93
Lm75write = &H92
Call Read_temp
Buffer = "<br></b>Temperature 2 <b><!--T2-->" + Chr(vz) + Str(temp_vk) + "." + Chr(temp_nk) + " C"
End If
And here the
subroutines used
Sub Read_temp
I2cstart
'LM75 address on the bus
I2cwbyte Lm75read
'Read Temp register
I2crbyte Msb , Ack
'before decimal point
I2crbyte Lsb , Nack
'after decimal point
I2cstop
Call 2er_in_dec
Call Check
Call Resetpointer
End Sub
' *** 2e complement in decimal ***
Sub 2er_in_dec
If Msb.7 = 0 Then
'Check of temp positive Bit7 = 0
Temp_vk = Msb
Else
'else negative
Temp_vk = Not Msb
'ignore
End If
End Sub
' *** Decimal 2e complement ***
Sub Dec_in_2er
Msb = Not Msb
'invert
Msb = Msb + 1
'add 1
End Sub
' *** check on I2C Bus error ***
Sub Resetpointer
If Err = 1 Then
Print "I²C Bus Error " ; Err
End If
I2cstart
I2cwbyte Lm75write
I2cwbyte &B00000000 , Nack
I2cstop
If Err = 1 Then
Print "Resetpointer - I²C Bus Error "
End If
End Sub
' ***
Sub Check
' *** check on positive ***
If Msb.7 = 1 Then
Vz = " "
Temp_vk = Makeint(msb , &HFF)
Else
Temp_vk = Makeint(msb , &H00)
' positive
End If
' *** check if temp negative and 0,5 °C bit set ***
If Lsb.7 = 1 And Msb.7 = 1 Then
Incr Temp_vk
'incr. value
End If
' *** check if temp <> 0 and positive
If Msb <> 0 And Msb.7 = 0 Then
Vz = "+" 'Dann "+" Zeichen
End If
' *** check if temp = -0,5°C ***
If Msb = 255 And Lsb = 255 Then
' -0,5 °Grad corr.
Vz = "-"
End If
' *** check on exact 0°C ***
If Msb = 0 And Lsb.7 = 0 Then
'on 0 ° C
Vz = " "
End If
' ** after decimal point ***
If Lsb.7 = 1 Then
'after dec. point = ,5 ?
Temp_nk = "5"
Else
Temp_nk = "0"
End If
End Sub
Add two commands in the
HTML-data lines on separate lines (just like %MRELAIS1%, %IP%, %END%
etc.).
Data "%TEMP1%"
Data "%TEMP2%"
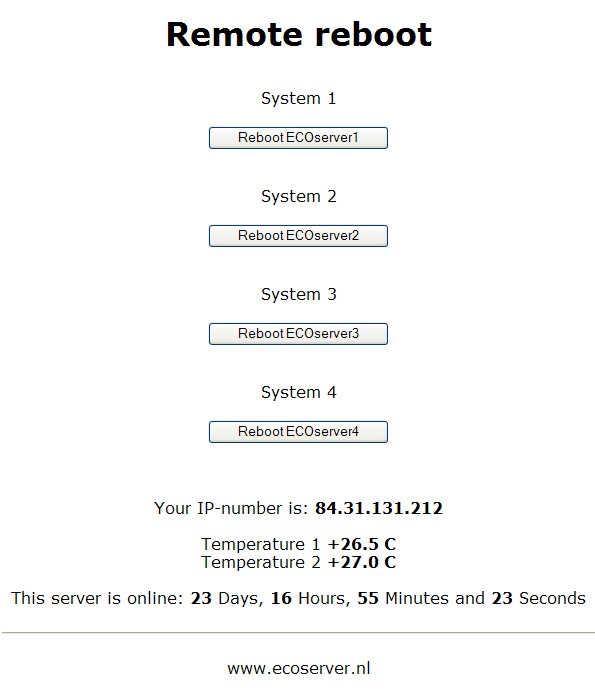
It will end up like this
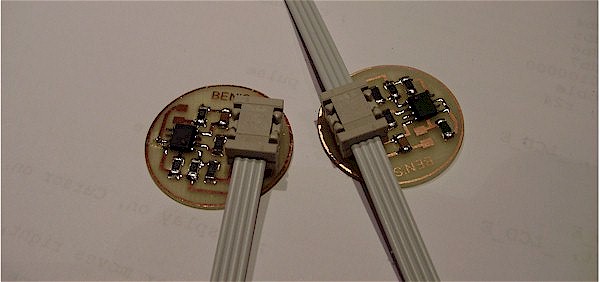
Prototypes
of the LM75 temperaturesensors.
A scan of the I2c-bus
and on &H90 and &H94 the two sensors can be found.
And here the Bascom-AVR
code. What are the differences?
Removed the global login-screen because there were problems with
Internet Explorer 7 and Firefox, password possible on all buttons, two
temperature-sensors and a username/password on the configuration screen.
Quote("test")?
I compile it and it is
giving errors about a Quote-command?
Yes, that is a new command and it will be released in the next
Bascom-AVR version. Instead
of a chr(34) + "test"+ chr(34)
you can use quote("test")
to get the same.
Makes the HTML-code more readable.
red_bokito_260908_0000.txt
and here the compiled
files red_bokito_longboard_260908_0000.hex red_bokito_smallboard_260908_0000.hex
To get in the
configuration: user:admin password:fire
|
Monitoring
the temperatures with HTTP-GET... |
It is possible to read the temperatures
and switch the relays from within a external program, not using a
browser. Post_demo is such a external program. When doing a GET on your
board you will get this:
HTTP/1.0 200 Document follows
Server: ecoserver
Content-Type: text/html
<html><head><meta http-equiv="Pragma" Content="no
cache"><title>Remote reboot</title></head><body><center><table width="600"><tr><td align="center"><font face="verdana" color="#000000"><H1>Remote reboot</H1><br>System 1<br><form action="/" method="POST"><input type="hidden" name="MRELAIS1" value="0"><input type = "submit" value = "Reboot ECOserver1"></form><br>System 2<br><form action="/" method="POST"><input type="hidden" name="MRELAIS2" value="0"><input type = "submit" value = "Reboot ECOserver2"></form><br>System 3<br><form action="/" method="POST"><input type="hidden" name="MRELAIS3" value="0"><input type = "submit" value = "Reboot ECOserver3"></form><br>System 4<br/><form action="/" method="POST"><input type="hidden" name="MRELAIS4" value="0"><input type = "submit" value = "Reboot ECOserver4"></form><br><br><font face="verdana" color="#000000">Your IP-number is: <b>84.31.131.212</b><br><br>Temperature 1 <b><!--T1-->+26.5 C<br></b>Temperature 2 <b><!--T2-->+27.0 C<br><br></b>This server is online: <b>23</b> Days, <b>17</b> Hours, <b>8</b> Minutes and <b>11</b> Seconds<br><br><hr><br>www.ecoserver.nl</a></font></td></tr></table></center></body></html>
I have added two remarks in the
HTML-code to make it easy just to pick up both temperatures.
If you want to test it,
you can download a Visual Basic 6.0 version of the HTTP-GET-program
here. You should put the IP-number of your board in it and the request
mode on GET. You can also switch the relais by putting the variables
MRELAIS1 - MRELAIS4 with a value 0 in it. Request mode on POST.
It should be possible
to poll several boards in a datacenter and give an alarm when
temperatures are going over a pre-defined threshold.
post-demo.zip
|
Remote
ADC monitoring.... |
Someone asked if I could monitor voltage over the Internet. The
Atmega168 has 6 ADC channels so that wouldn't be that hard. Also Thomas
Heldt has used ADC in his examples.
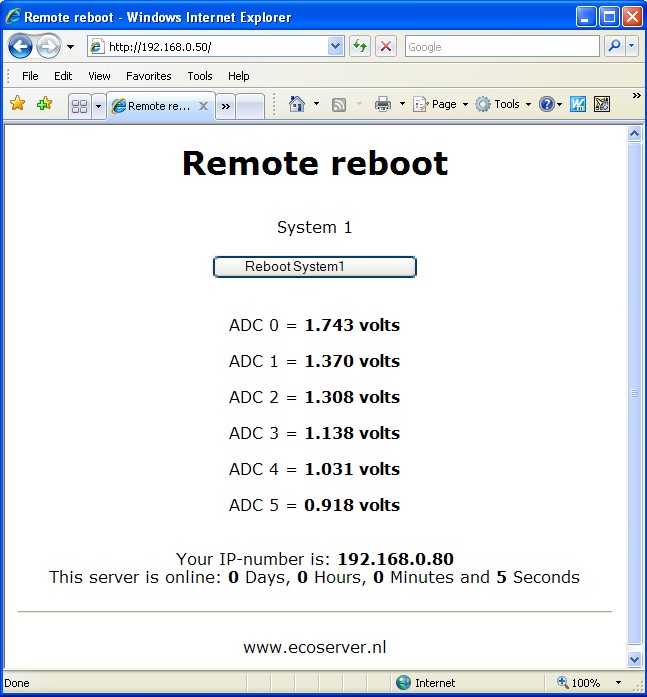
A total of 6 ADC lines
can be used.
And here some additions
to the Red Bokito code
Configure ADC on the
Atmega168
Config Adc = Single , Prescaler = Auto , Reference = Avcc
Start
ADC
Adding
extra commands. You have to make this 6 times if you want to use all
channels.
If Buffer = "%ADC0%" Then
Adctemp = Getadc(0)
Adcref2 = Adcref / 1024
Adcresult = Adcref2 * Adctemp
Buffer = Fusing(adcresult , "#.###")
End If
HTML-datalines with the
ADC-commands
Data "</b><br>ADC 0 = <b>"
Data "%ADC0%"
Data " volts</b><br>"
Data "</b><br>ADC 1 = <b>"
Data "%ADC1%"
Data " volts</b><br>"
Data "</b><br>ADC 2 = <b>"
Data "%ADC2%"
Data " volts</b><br>"
Data "</b><br>ADC 3 = <b>"
Data "%ADC3%"
Data " volts</b><br>"
Data "</b><br>ADC 4 = <b>"
Data "%ADC4%"
Data " volts</b><br>"
Data "</b><br>ADC 5 = <b>"
Data "%ADC5%"
Data " volts</b><br>"
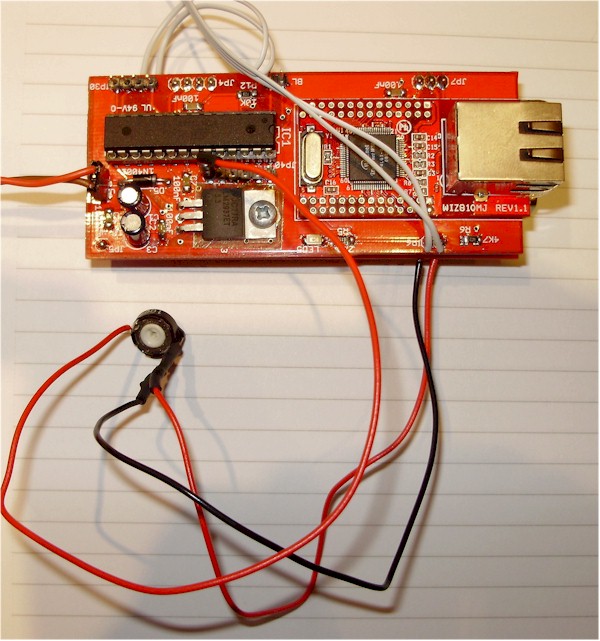
Measuring the voltages
over a potentiometer. On a single ADC-line.
|
Switching
the relais with a Visual Basic program |
Switching the Red Bokito from the command line
or with a Visual Basic program. With one extra component (Microsoft
Internet Transfer Control 6.0) and four buttons it is possible to switch
the relays from the Red Bokito direct from within a Visual Basic
program, or compiled to an EXE-file with one command with some
parameters.
Start a new form, and at the components
add Microsoft Internet Transfer Control 6.0. Put this control on the
form and name is inet1
Put four buttons on the form and add
the code just like in the picture below.
Run it. When the Red Bokito is
configured without using passwords on the buttons the relays and the LED
should go on for half a second.
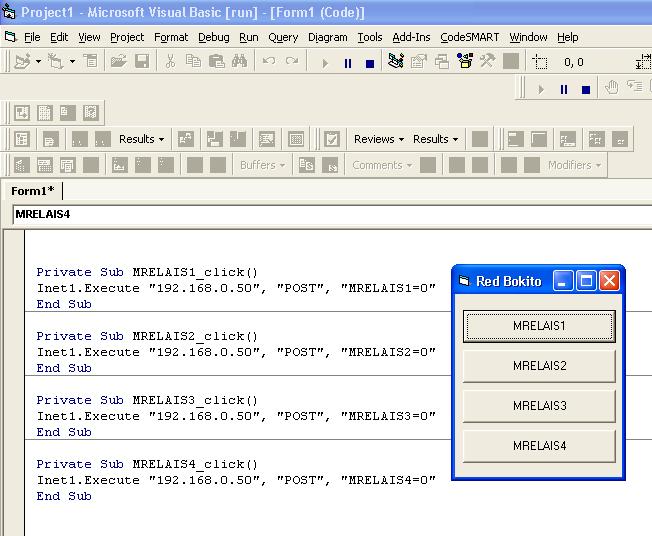
And here a command line
version
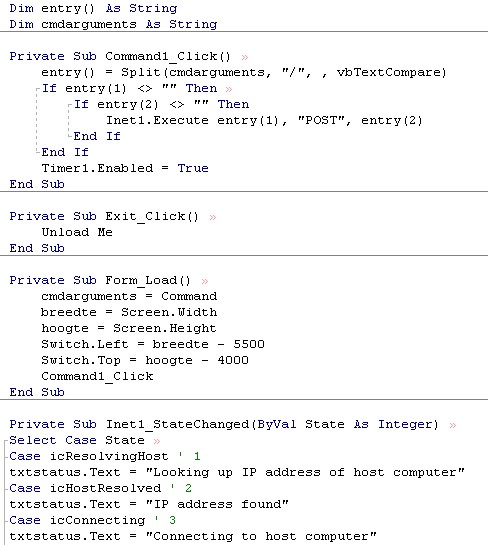
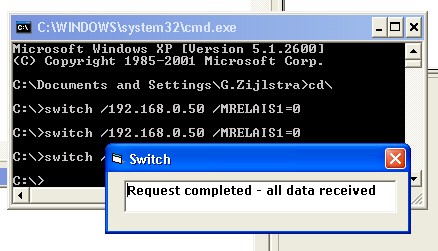
|
Another
example, reading 8 opto-couplers over the Internet.... |
Prototype
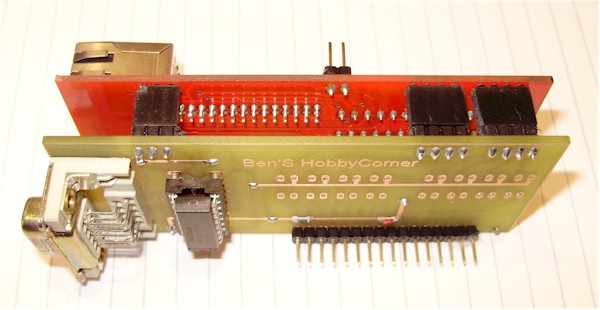
The optocouplers still
have to be fitted in these pictures. PC817 will be used.
And here the commercial
version in some nice cases





 ABS-71
Hammond 1594 C
|
Email-switcher with confirmation |
I would like to switch the relays
by email? That is possible. Here an example of using POP (Post Office
Protocol) to check mail, and SMTP to send mail. I am using two separate
sockets. It is possible to combine some subroutines but for clarity, I
copied
and changed two of the major subroutines.
'----------------------------------------------------------------------------------------
' Atmega168 and WIZ810MJ All you need to get a email-switch working - POP / SMTP
'----------------------------------------------------------------------------------------
'
$regfile = "m168def.dat"
$crystal = 8000000
$baud = 9600
'you should keep the stack-settings this high, if you make them smaller strange things can happen.
$hwstack = 128
$swstack = 128
$framesize = 128
$include "w5100.inc"
Dim Value As Byte
Dim Adres As Word
Dim Adresl As Byte At Adres Overlay
Dim Adresh As Byte At Adres + 1 Overlay
Dim Pointer As Word
Dim Pointerl As Byte At Pointer Overlay
Dim Pointerh As Byte At Pointer + 1 Overlay
Dim Size As Word
Dim Sizel As Byte At Size Overlay
Dim Sizeh As Byte At Size + 1 Overlay
Dim Bpointer As Word
Dim X As Word
Dim Freesize As Word
Dim Sendsize As Integer
Dim Tmp_str As String * 1
Dim Tmp_value As Byte
Dim Startpos As Word
Dim Tx_wr As Word
Dim Offset As Word
Dim Offsend As Word
Dim Uppersize As Word
Dim Startadress As Word
Dim Highbyte As Byte
Dim Lowbyte As Byte
Dim Buffer As String * 180
Dim Glaenge As Word
Dim Hulp As Byte
Dim Parameter As String * 3
Dim Reaction As String * 20
Dim Preaction As Byte
Dim Timeout As Bit
Dim Linebuffer As String * 80
Dim Frombuffer As String * 80
Dim Tobuffer As String * 80
Dim Subjectbuffer As String * 80
Dim Pincode As String * 4
Dim Begin As Byte
Dim Eind As Byte
Dim Email As String * 30
Dim Linefeed_flag As Byte
Dim Bcount As Byte
Dim Ar(3) As String * 8
' 0 = long board
' 1 = sandwichboard
Const Board = 1
'Used Wiz5100 ports and pins
Wiz5100_cs Alias Portb.2 'Chipselect WIZ810MJ
Print "Start mail-retriever.."
Wait 1
'You can still choose the small or the long board
#if Board 'sandwichboard
Wiz5100_res Alias Portd.3 'reset of WIZ810MJ
'Other used ports and pins
Relais1 Alias Portd.4
Relais2 Alias Portd.5
Relais3 Alias Portd.6
Relais4 Alias Portd.7
#elseif
Wiz5100_res Alias Portc.0 'long board
Relais1 Alias Portc.1
Relais2 Alias Portc.2
Relais3 Alias Portc.3
Relais4 Alias Portc.4
#endif
'Used ports and pins
Config Wiz5100_cs = Output
Config Wiz5100_res = Output
'Config the port/pin directions
Config Relais1 = Output
Config Relais2 = Output
Config Relais3 = Output
Config Relais4 = Output
Relais1 = 1
Relais2 = 1
Relais3 = 1
Relais4 = 1
'Configuration of the SPI-bus
Config Spi = Hard , Interrupt = Off , Data Order = Msb , Master = Yes , Polarity = Low , _
Phase = 0 , Clockrate = 4 , Noss = 0
'Init the spi pins
Spiinit
Wait 1
'Up to here nothing new, perhaps some new variables
'And here the declares of the subroutines. S0 for Socket 0, S1 for Socket 1
'Here we declare the used sub routines
Declare Sub Wiz5100_init
Declare Sub Wiz5100_readvalue(byval Reg As Word)
Declare Sub Wiz5100_writevalue(byval Reg As Word , Byval Value As Byte)
Declare Sub Wiz5100_reset
Declare Sub Wiz5100_s0_sendit
Declare Sub Wiz5100_s0_waitfor(byval Parameter As String)
Declare Sub Wiz5100_s1_sendit
Declare Sub Wiz5100_s1_waitfor(byval Parameter As String)
Declare Sub Catchlines
Declare Sub Confirmmail(byval Frombuffer As String , Byval Subjectbuffer As String)
Declare Sub Mrelais1_toggle
Declare Sub Mrelais2_toggle
Declare Sub Mrelais3_toggle
Declare Sub Mrelais4_toggle
Declare Sub Mrelais1_on
Declare Sub Mrelais2_on
Declare Sub Mrelais3_on
Declare Sub Mrelais4_on
Declare Sub Mrelais1_off
Declare Sub Mrelais2_off
Declare Sub Mrelais3_off
Declare Sub Mrelais4_off
Dim Wiz5100_opcode_read As Byte
Wiz5100_opcode_read = 15
Dim Wiz5100_opcode_write As Byte
Wiz5100_opcode_write = 240
Call Wiz5100_init 'We initialize the Wiz810MJ
Do
Print "POP Mail checker"
'socket 0 - retrieve email
'protocol = tcp
Call Wiz5100_writevalue(w5100_s0_mr , Sn_mr_tcp)
Call Wiz5100_writevalue(w5100_s0_port0 , &H13) 'port 5000
Call Wiz5100_writevalue(w5100_s0_port1 , &H88)
'here the IP-number and port to read the email (POP)
Call Wiz5100_writevalue(w5100_s0_dipr0 , 91) 'IP-number of mail-server
Call Wiz5100_writevalue(w5100_s0_dipr1 , 198)
Call Wiz5100_writevalue(w5100_s0_dipr2 , 169)
Call Wiz5100_writevalue(w5100_s0_dipr3 , 81)
Call Wiz5100_writevalue(w5100_s0_dport0 , 00) 'port 110
Call Wiz5100_writevalue(w5100_s0_dport1 , 110)
'give the open command
Call Wiz5100_writevalue(w5100_s0_cr , Sn_cr_open)
'wait time to get ARP-request finished
Wait 2
Do
Call Wiz5100_readvalue(w5100_s0_sr)
'sock init?
Loop Until Value = Sock_init
'give the connect command
Call Wiz5100_writevalue(w5100_s0_cr , Sn_cr_connect)
Call Wiz5100_readvalue(w5100_s0_ir)
If Value.3 = 1 Then
Print "Timeout"
Timeout = 1
Goto Quit_s0
End If
Wait 2
Do
Call Wiz5100_readvalue(w5100_s0_sr)
'Sock Established
Loop Until Value = Sock_established
Call Wiz5100_s0_waitfor( "+OK")
If Timeout = 1 Then Goto Quit_s0
'to keep standard things like username, password etc. outside the program you can find them as
'DATA-lines at the end of the program
'Here we start the conversation
Restore User
Read Buffer
Buffer = "USER " + Buffer + Chr(13) + Chr(10)
Call Wiz5100_s0_sendit
Call Wiz5100_s0_waitfor( "+OK")
If Timeout = 1 Then Goto Quit_s0
Restore Password
Read Buffer
Buffer = "PASS " + Buffer + Chr(13) + Chr(10)
Call Wiz5100_s0_sendit
Call Wiz5100_s0_waitfor( "+OK")
If Timeout = 1 Then Goto Quit_s0
'In another example I have used LIST, now I am using STAT to check how many emails are in the queue
Buffer = "STAT" + Chr(13) + Chr(10)
Call Wiz5100_s0_sendit
Call Wiz5100_s0_waitfor( "+OK")
If Timeout = 1 Then Goto Quit_s0
Bcount = Split(reaction , Ar(1) , " ")
Print
Print "You have got " ; Ar(2) ; " message"
Hulp = Val(ar(2))
If Hulp > 1 Then
Print "s"
End If
'In ar(2) the number of emails. Get the first one.
If Val(ar(2)) > 0 Then
Buffer = "RETR 1" + Chr(13) + Chr(10)
Call Wiz5100_s0_sendit
Call Wiz5100_s0_waitfor( "+OK")
If Timeout = 1 Then Goto Quit_s0
Buffer = "DELE 1" + Chr(13) + Chr(10)
Call Wiz5100_s0_sendit
Call Wiz5100_s0_waitfor( "+OK")
If Timeout = 1 Then Goto Quit_s0
End If
Quit_s0:
Buffer = "quit" + Chr(13) + Chr(10)
Call Wiz5100_s0_sendit
'Set Discon Flag
Call Wiz5100_writevalue(w5100_s0_cr , &H8)
'When we arrived here we got the email, got a few strings with all details like Pincode
'(Accesscode), from, to and subject.
Pincode = ""
If Frombuffer <> "" Then
Print Frombuffer
Print Tobuffer
Print Subjectbuffer
Restore Pincode
Read Buffer
If Mid(subjectbuffer , 10 , 4) <> Buffer Then
Subjectbuffer = "Wrong code"
Print "Wrong accescode"
Pincode = "*"
End If
Print "POP Mail checker"
Print Mid(subjectbuffer , 10)
Frombuffer = Mid(frombuffer , 7)
Subjectbuffer = Mid(subjectbuffer , 15)
Hulp = Len(subjectbuffer)
Decr Hulp
Subjectbuffer = Left(subjectbuffer , Hulp)
Select Case Subjectbuffer
Case "RELAY1=ON" : Call Mrelais1_on
Case "RELAY2=ON" : Call Mrelais2_on
Case "RELAY3=ON" : Call Mrelais3_on
Case "RELAY4=ON" : Call Mrelais4_on
Case "RELAY1=OFF" : Call Mrelais1_off
Case "RELAY2=OFF" : Call Mrelais2_off
Case "RELAY3=OFF" : Call Mrelais3_off
Case "RELAY4=OFF" : Call Mrelais4_off
'RELAY1=TOGGLE
Case "RELAY1=TOGGLE" : Call Mrelais1_toggle
Case "RELAY2=TOGGLE" : Call Mrelais2_toggle
Case "RELAY3=TOGGLE" : Call Mrelais3_toggle
Case "RELAY4=TOGGLE" : Call Mrelais4_toggle
Case Else : Subjectbuffer = "Unknown command" + Subjectbuffer
End Select
If Pincode = "*" Then
Subjectbuffer = "Wrong accesscode"
End If
Call Confirmmail(frombuffer , Subjectbuffer)
Tobuffer = ""
Frombuffer = ""
Subjectbuffer = ""
End If
Wait 120
Cls
Home
Loop
End
Sub Wiz5100_init
Call Wiz5100_reset 'Hardware reset
'Register reset
Call Wiz5100_writevalue(w5100_mr , &H80)
'Set gateway IP adress
Call Wiz5100_writevalue(w5100_gar0 , 192) '192.168.0.254 My gateway
Call Wiz5100_writevalue(w5100_gar1 , 168)
Call Wiz5100_writevalue(w5100_gar2 , 0)
Call Wiz5100_writevalue(w5100_gar3 , 254)
'Set Subnetmask
Call Wiz5100_writevalue(w5100_subr0 , 255) '255.255.255.0 networkmask
Call Wiz5100_writevalue(w5100_subr1 , 255)
Call Wiz5100_writevalue(w5100_subr2 , 255)
Call Wiz5100_writevalue(w5100_subr3 , 0)
'Set MAC
Call Wiz5100_writevalue(w5100_shar0 , &H00) 'MAC-address
Call Wiz5100_writevalue(w5100_shar1 , &H10)
Call Wiz5100_writevalue(w5100_shar2 , &H20)
Call Wiz5100_writevalue(w5100_shar3 , &H30)
Call Wiz5100_writevalue(w5100_shar4 , &H40)
Call Wiz5100_writevalue(w5100_shar5 , &H50)
'Set own IP adress
Call Wiz5100_writevalue(w5100_sipr0 , 192) 'IP-number of device
Call Wiz5100_writevalue(w5100_sipr1 , 168)
Call Wiz5100_writevalue(w5100_sipr2 , 0)
Call Wiz5100_writevalue(w5100_sipr3 , 75)
'Assign 4k memory to socket 0 and socket 1 for RX
Call Wiz5100_writevalue(w5100_rmsr , &B00001010)
'Assign 4k memory to socket 0 and socket 1 for TX
Call Wiz5100_writevalue(w5100_tmsr , &B00001010)
End Sub
Sub Wiz5100_readvalue(reg)
Adres = Reg
Reset Wiz5100_cs
Spiout Wiz5100_opcode_read , 1
Spiout Adresh , 1
Spiout Adresl , 1
Spiin Value , 1
Set Wiz5100_cs
End Sub
Sub Wiz5100_writevalue(reg , Value )
Adres = Reg
Reset Wiz5100_cs
Spiout Wiz5100_opcode_write , 1
Spiout Adresh , 1
Spiout Adresl , 1
Spiout Value , 1
Set Wiz5100_cs
End Sub
Sub Wiz5100_reset
Waitms 250
Wiz5100_res = 1
Waitms 250
Wiz5100_res = 0
Waitms 250
Wiz5100_res = 1
End Sub
'There are two almost identical routines to send things to the W5100, one for socket 0 and
'one for socket 1
Sub Wiz5100_s0_sendit
Sendsize = Len(buffer)
Freesize_s0:
Call Wiz5100_readvalue(w5100_s0_tx_fsr0)
Freesize = Value
Shift , Freesize , Left , 8
Call Wiz5100_readvalue(w5100_s0_tx_fsr1)
Freesize = Freesize + Value
If Freesize < Sendsize Then
Goto Freesize_s0
End If
Call Wiz5100_readvalue(w5100_s0_tx_wr0)
Tx_wr = Value
Shift , Tx_wr , Left , 8
Call Wiz5100_readvalue(w5100_s0_tx_wr1)
Tx_wr = Tx_wr + Value
Startpos = Tx_wr
Offset = Tx_wr And &H0FFF
Startadress = &H4000 + Offset
Offsend = Offset + Sendsize
Pointer = Startadress
If Offsend > &H1000 Then
Uppersize = &H1000 - Offset
For X = 1 To Uppersize
Tmp_str = Mid(buffer , X , 1)
Tmp_value = Asc(tmp_str)
Call Wiz5100_writevalue(pointer , Tmp_value)
Incr Pointer
Next X
Pointer = &H4000
Incr Uppersize
For X = Uppersize To Sendsize
Tmp_str = Mid(buffer , X , 1)
Tmp_value = Asc(tmp_str)
Call Wiz5100_writevalue(pointer , Tmp_value)
Incr Pointer
Next X
Else
For X = 1 To Sendsize
Tmp_str = Mid(buffer , X , 1)
Tmp_value = Asc(tmp_str)
Call Wiz5100_writevalue(pointer , Tmp_value)
Incr Pointer
Next X
End If
Call Wiz5100_readvalue(w5100_s0_tx_wr0)
Startpos = Value
Shift , Startpos , Left , 8
Call Wiz5100_readvalue(w5100_s0_tx_wr1)
Startpos = Startpos + Value
Glaenge = Startpos + Sendsize
'Send text
Highbyte = High(glaenge)
Call Wiz5100_writevalue(w5100_s0_tx_wr0 , Highbyte)
Lowbyte = Low(glaenge)
Call Wiz5100_writevalue(w5100_s0_tx_wr1 , Lowbyte)
'Set SEND flag
Call Wiz5100_writevalue(w5100_s0_cr , Sn_cr_send)
End Sub
'get reaction of the mailserver
Sub Wiz5100_s0_waitfor(byval Parameter As String)
Timeout = 0
Do
'check on RECV
Call Wiz5100_readvalue(w5100_s0_ir)
If Value.3 = 1 Then
Print "Timeout"
Timeout = 1
Exit Sub
End If
Loop Until Value.2 = 1
Wait 1 ' important to get the whole message
Reaction = ""
Preaction = 0
Call Wiz5100_readvalue(w5100_s0_rx_rd0)
Pointerh = Value
Call Wiz5100_readvalue(w5100_s0_rx_rd1)
Pointerl = Value
'Socket 0 RX Received Size
Call Wiz5100_readvalue(w5100_s0_rx_rsr0)
Sizeh = Value
Call Wiz5100_readvalue(w5100_s0_rx_rsr1)
Sizel = Value
Startpos = Pointer
Offset = Startpos And &H0FFF
Startadress = &H6000 + Offset
Offsend = Offset + Size
Bpointer = Startadress
If Offsend > &H1000 Then
Uppersize = &H1000 - Offset
For X = 1 To Uppersize
Call Wiz5100_readvalue(bpointer)
Call Catchlines
Print Chr(value) ;
If Preaction < 20 Then
Reaction = Reaction + Chr(value)
Incr Preaction
End If
Incr Bpointer
Next X
Bpointer = &H6000
Incr Uppersize
For X = Uppersize To Size
Call Wiz5100_readvalue(bpointer)
Call Catchlines
Print Chr(value) ;
If Preaction < 20 Then
Reaction = Reaction + Chr(value)
Incr Preaction
End If
Incr Bpointer
Next X
Else
For X = 1 To Size
Call Wiz5100_readvalue(bpointer)
Call Catchlines
Print Chr(value) ;
If Preaction < 20 Then
Reaction = Reaction + Chr(value)
Incr Preaction
End If
Incr Bpointer
Next X
End If
Print
Pointer = Pointer + Size
'update pointer
Call Wiz5100_writevalue(w5100_s0_rx_rd0 , Pointerh)
Call Wiz5100_writevalue(w5100_s0_rx_rd1 , Pointerl)
Call Wiz5100_writevalue(w5100_s0_cr , Sn_cr_recv)
'reset interrupt
Call Wiz5100_writevalue(w5100_s0_ir , &B100)
'
Call Wiz5100_readvalue(w5100_s0_cr)
If Parameter = "???" Then
Print Reaction
Exit Sub
End If
If Parameter <> Left(reaction , 3) Then
Print "Unexpected reaction from mailserver " ; Reaction
Goto Quit_s0
End If
End Sub
'Here we catch the To, From, Subject lines. Catching lines from linefeed to linefeed and comparing them
'with the keywords we must have.
Sub Catchlines
' Get lines From:
' To:
' Subject:
'
If Value = &H0A Then
Linefeed_flag = 1
If Left(linebuffer , 6) = "From: " Then
Frombuffer = Linebuffer
End If
If Left(linebuffer , 4) = "To: " Then
Tobuffer = Linebuffer
End If
If Left(linebuffer , 9) = "Subject: " Then
Subjectbuffer = Linebuffer
End If
Linebuffer = ""
Exit Sub
End If
If Linefeed_flag = 1 Then
Linebuffer = Linebuffer + Chr(value)
If Len(linebuffer) > 79 Then
Linebuffer = ""
End If
End If
End Sub
'Here the subroutine to send a confirmation email. SMTP is used.
'With Base64 encrypted USER and PASSWORD.
Sub Confirmmail(byval Frombuffer , Byval Subjectbuffer)
' socket 1 - SMTP send confirmation mail
Print "Message to: " ; Frombuffer
Print "Subject: " ; Subjectbuffer
'filter just the email-adres
Begin = Instr(frombuffer , "<")
Begin = Begin + 1
Eind = Instr(frombuffer , ">")
Hulp = Eind - Begin
Email = Mid(frombuffer , Begin , Hulp)
Print "Email to " ; Email
Print "SMTP confirm"
'protocol = tcp
Call Wiz5100_writevalue(w5100_s1_mr , Sn_mr_tcp)
Call Wiz5100_writevalue(w5100_s1_port0 , &H13) 'port 5000
Call Wiz5100_writevalue(w5100_s1_port1 , &H88)
'Here the IP-number and Port of my provider
Call Wiz5100_writevalue(w5100_s1_dipr0 , 195) 'IP-number of mail-server
Call Wiz5100_writevalue(w5100_s1_dipr1 , 47)
Call Wiz5100_writevalue(w5100_s1_dipr2 , 247)
Call Wiz5100_writevalue(w5100_s1_dipr3 , 201)
Call Wiz5100_writevalue(w5100_s1_dport0 , &H09) 'port 2525
Call Wiz5100_writevalue(w5100_s1_dport1 , &HDD)
'give the open command
Call Wiz5100_writevalue(w5100_s1_cr , Sn_cr_open)
'wait time to get ARP-request finished
Wait 2
Do
Call Wiz5100_readvalue(w5100_s1_sr)
'sock init?
Loop Until Value = Sock_init
'give the connect command
Call Wiz5100_writevalue(w5100_s1_cr , Sn_cr_connect)
Call Wiz5100_readvalue(w5100_s1_ir)
If Value.3 = 1 Then
Print "Timeout"
Timeout = 1
Goto Quit_s1
End If
Wait 2
Do
Call Wiz5100_readvalue(w5100_s1_sr)
'Sock Established
Loop Until Value = Sock_established
Call Wiz5100_s1_waitfor( "220")
If Timeout = 1 Then Goto Quit_s1
'Yes, it is a fake domainname
Buffer = "HELO sokophouder.nl" + Chr(13) + Chr(10)
Call Wiz5100_s1_sendit
Call Wiz5100_s1_waitfor( "250")
If Timeout = 1 Then Goto Quit_s1
Buffer = "AUTH LOGIN"+ Chr(13) + Chr(10)
Call Wiz5100_s1_sendit
Call Wiz5100_s1_waitfor( "334")
If Timeout = 1 Then Goto Quit_s1
'Here the Base64-encrypted username is fetched. See DATA-lines belowe.
Restore User_bs64
Read Buffer
Buffer = Buffer + Chr(13) + Chr(10)
Call Wiz5100_s1_sendit
Call Wiz5100_s1_waitfor( "334")
If Timeout = 1 Then Goto Quit_s1
Restore Password_bs64
Read Buffer
Buffer = Buffer + Chr(13) + Chr(10)
Call Wiz5100_s1_sendit
Call Wiz5100_s1_waitfor( "235")
If Timeout = 1 Then Goto Quit_s1
Buffer = "MAIL FROM: alarm@sokophouder.nl" + Chr(13) + Chr(10)
Call Wiz5100_s1_sendit
Call Wiz5100_s1_waitfor( "250")
If Timeout = 1 Then Goto Quit_s1
Buffer = "RCPT TO: " + Email + Chr(13) + Chr(10)
Call Wiz5100_s1_sendit
Call Wiz5100_s1_waitfor( "250")
If Timeout = 1 Then Goto Quit_s1
Buffer = "DATA" + Chr(13) + Chr(10)
Call Wiz5100_s1_sendit
Call Wiz5100_s1_waitfor( "354")
If Timeout = 1 Then Goto Quit_s1
Buffer = "From: Alarm@sokophouder.nl" + Chr(13) + Chr(10)
Buffer = Buffer + "To: " + Email + Chr(13) + Chr(10)
Buffer = Buffer + "Subject: Confirm " + Subjectbuffer + Chr(13) + Chr(10)
Call Wiz5100_s1_sendit
Wait 1
Buffer = "." + Chr(13) + Chr(10)
Call Wiz5100_s1_sendit
Call Wiz5100_s1_waitfor( "250")
If Timeout = 1 Then Goto Quit_s1
Quit_s1:
Buffer = "quit" + Chr(13) + Chr(10)
Call Wiz5100_s1_sendit
'Set Discon Flag
Call Wiz5100_writevalue(w5100_s1_cr , &H8)
Print "POP Mail checker"
End Sub
'***** socket 1
Sub Wiz5100_s1_sendit
Sendsize = Len(buffer)
Freesize_s1:
Call Wiz5100_readvalue(w5100_s1_tx_fsr0)
Freesize = Value
Shift , Freesize , Left , 8
Call Wiz5100_readvalue(w5100_s1_tx_fsr1)
Freesize = Freesize + Value
If Freesize < Sendsize Then
Goto Freesize_s1
End If
Call Wiz5100_readvalue(w5100_s1_tx_wr0)
Tx_wr = Value
Shift , Tx_wr , Left , 8
Call Wiz5100_readvalue(w5100_s1_tx_wr1)
Tx_wr = Tx_wr + Value
Startpos = Tx_wr
Offset = Tx_wr And &H0FFF
Startadress = &H5000 + Offset
Offsend = Offset + Sendsize
Pointer = Startadress
If Offsend > &H1000 Then
Uppersize = &H1000 - Offset
For X = 1 To Uppersize
Tmp_str = Mid(buffer , X , 1)
Tmp_value = Asc(tmp_str)
Call Wiz5100_writevalue(pointer , Tmp_value)
Incr Pointer
Next X
Pointer = &H5000
Incr Uppersize
For X = Uppersize To Sendsize
Tmp_str = Mid(buffer , X , 1)
Tmp_value = Asc(tmp_str)
Call Wiz5100_writevalue(pointer , Tmp_value)
Incr Pointer
Next X
Else
For X = 1 To Sendsize
Tmp_str = Mid(buffer , X , 1)
Tmp_value = Asc(tmp_str)
Call Wiz5100_writevalue(pointer , Tmp_value)
Incr Pointer
Next X
End If
Call Wiz5100_readvalue(w5100_s1_tx_wr0)
Startpos = Value
Shift , Startpos , Left , 8
Call Wiz5100_readvalue(w5100_s1_tx_wr1)
Startpos = Startpos + Value
Glaenge = Startpos + Sendsize
'Send text
Highbyte = High(glaenge)
Call Wiz5100_writevalue(w5100_s1_tx_wr0 , Highbyte)
Lowbyte = Low(glaenge)
Call Wiz5100_writevalue(w5100_s1_tx_wr1 , Lowbyte)
'Set SEND flag
Call Wiz5100_writevalue(w5100_s1_cr , Sn_cr_send)
End Sub
'get reaction of the mailserver
Sub Wiz5100_s1_waitfor(byval Parameter As String)
Timeout = 0
Do
'check on RECV
Call Wiz5100_readvalue(w5100_s1_ir)
If Value.3 = 1 Then
Print "Timeout"
Timeout = 1
Exit Sub
End If
Loop Until Value.2 = 1
Wait 1 ' important to get the whole message!!
Reaction = ""
Preaction = 0
Call Wiz5100_readvalue(w5100_s1_rx_rd0)
Pointerh = Value
Call Wiz5100_readvalue(w5100_s1_rx_rd1)
Pointerl = Value
'Socket 1 RX Received Size
Call Wiz5100_readvalue(w5100_s1_rx_rsr0)
Sizeh = Value
Call Wiz5100_readvalue(w5100_s1_rx_rsr1)
Sizel = Value
Startpos = Pointer
Offset = Startpos And &H0FFF
Startadress = &H7000 + Offset
Offsend = Offset + Size
Bpointer = Startadress
If Offsend > &H1000 Then
Uppersize = &H1000 - Offset
For X = 1 To Uppersize
Call Wiz5100_readvalue(bpointer)
Call Catchlines
Print Chr(value) ;
If Preaction < 20 Then
Reaction = Reaction + Chr(value)
Incr Preaction
End If
Incr Bpointer
Next X
Bpointer = &H7000
Incr Uppersize
For X = Uppersize To Size
Call Wiz5100_readvalue(bpointer)
Call Catchlines
Print Chr(value) ;
If Preaction < 20 Then
Reaction = Reaction + Chr(value)
Incr Preaction
End If
Incr Bpointer
Next X
Else
For X = 1 To Size
Call Wiz5100_readvalue(bpointer)
Call Catchlines
Print Chr(value) ;
If Preaction < 20 Then
Reaction = Reaction + Chr(value)
Incr Preaction
End If
Incr Bpointer
Next X
End If
Print
Pointer = Pointer + Size
'update pointer
Call Wiz5100_writevalue(w5100_s1_rx_rd0 , Pointerh)
Call Wiz5100_writevalue(w5100_s1_rx_rd1 , Pointerl)
Call Wiz5100_writevalue(w5100_s1_cr , Sn_cr_recv)
'reset interrupt
Call Wiz5100_writevalue(w5100_s1_ir , &B100)
'
Call Wiz5100_readvalue(w5100_s1_cr)
If Parameter = "???" Then
Print Reaction
Exit Sub
End If
If Parameter <> Left(reaction , 3) Then
Print "Unexpected reaction from mailserver " ; Reaction
Goto Quit_s1
End If
End Sub
'Here the subroutine to put the relais ON, OFF or TOGGLE them
Sub Mrelais1_toggle
Relais1 = 0
Waitms 250
Relais1 = 1
End Sub
Sub Mrelais2_toggle
Relais2 = 0
Waitms 250
Relais2 = 1
End Sub
Sub Mrelais3_toggle
Relais3 = 0
Waitms 250
Relais3 = 1
End Sub
Sub Mrelais4_toggle
Relais4 = 0
Waitms 250
Relais4 = 1
End Sub
Sub Mrelais1_on
Relais1 = 0
End Sub
Sub Mrelais2_on
Relais2 = 0
End Sub
Sub Mrelais3_on
Relais3 = 0
End Sub
Sub Mrelais4_on
Relais4 = 0
End Sub
Sub Mrelais1_off
Relais1 = 1
End Sub
Sub Mrelais2_off
Relais2 = 1
End Sub
Sub Mrelais3_off
Relais3 = 1
End Sub
Sub Mrelais4_off
Relais4 = 1
End Sub
User:
Data "alarm@sokophouder.nl"
'Have used the Bascom-AVR simulator to get the Base64-notation of the username.
'And the same for the Base64-password.
User_bs64:
Data "YWxhcm1Ac29rb3Bob3VkZXIubmw"
Password:
Data "hallodaar"
Password_bs64:
Data "aGFsbG9kYWFy"
Pincode:
Data "1956"
This is the way you should send PINCODE and the command. Because in the code you got a string from whom
the mail is, you could also allow just a few people to give commands and receive confirmation-emails.
And this is the confirmation mail you will receive.
Have added a small routine to delete mails with large attachments or large pieces of text in the
message part. Things like SPAM. In AR(3) the size of the mail is stored, is it larger that 4000 bytes
then it will be deleted.
DIM Whulp as word
If Val(ar(2)) > 0 Then
Print "Size of the mail is " ; Val(ar(3))
Whulp = Val(ar(3))
If Whulp > 4000 Then
Print "Delete large mail " ; Ar(3) ; " bytes"
Goto Skip_large_mails
End If
Buffer = "RETR 1" + Chr(13) + Chr(10)
Call Wiz5100_s0_sendit
Call Wiz5100_s0_waitfor( "+OK")
If Timeout = 1 Then Goto Quit_s0
Skip_large_mails:
Buffer = "DELE 1" + Chr(13) + Chr(10)
Call Wiz5100_s0_sendit
Call Wiz5100_s0_waitfor( "+OK")
If Timeout = 1 Then Goto Quit_s0
End If
The best way to work with this application is to use a dedicated email-addres. Will try to make an
application that checks for this kind of mails in your regulair mailbox and handle just those.
|
SNMP
Simple Network Management Protocol |
Add this
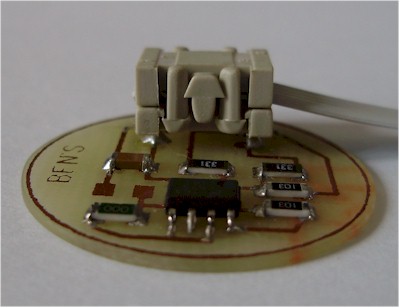
to get this
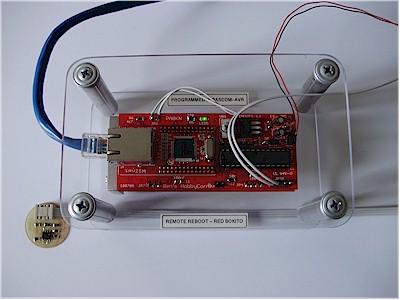
to catch this
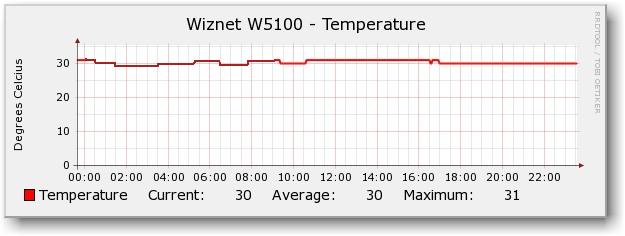
All done with SNMP.
What do you need to get this working on
one or more sensors, on one or more modules....
First, get a webserver running on a PC. The easiest way is to install
Wampserver, from www.wampserver.com
You get Apache, PHP and MySQL For Linux users, get LAMP
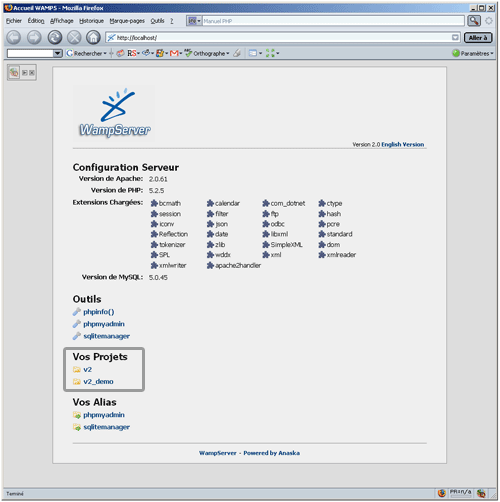
Get a copy of Cacti
www.cacti.net
There is a version for Windows and for
Linux
Get rrdtools, Net-SNMP, Spine and Cygwin.
Google for a installation-sheet for Cacti and you will see what to do.
And as last, download
APC_Environmental_Temp_sensor.1.5.zip
These are the templates we use to monitor
the Red Bokito with LM75-temperaturesensors.
Preliminary code...
My receive buffer starts at &H6000. My
transmit buffer starts at &H4000. Everytime I issue a Call Wiz5100_writevalue(W5100_s0_mr,
Sn_mr_udp) the read and write pointers are put back on their begin so
the SNMP string I receive can every time be found on exact the same
location in the receive-buffer and I can reply with an UDP-string at
exact the same spot in the transmit buffer.
I also use this on a TCP/IP
securitysystem, works very well...
About the code, everytime a SNMP call
is done, on port 161, with communitystring 'public' the Red Bokito will
return the temperature. Have to enhance the code to react on different
OID's and also implement SNMP-traps yet.
'---------------------------------------- -------
'
Atmega168 and WIZ810MJ - SNMP - working
'-----------------------------------------------
$regfile = "m168def.dat"
$crystal = 8000000
$baud = 9600
$hwstack = 128
$swstack
= 128
$framesize = 128
$include "w5100.inc"
Dim Value As Byte
Dim Adres As Word
Dim Adresl As Byte At Adres Overlay
Dim Adresh As Byte At Adres + 1 Overlay
Dim Src_ip(4) As Byte
Dim Src_prt(2) As Byte
Dim Wlength As Word
Dim Length(2) As Byte
Dim Wsrc_prt As Word
Dim Reqid(2) As Byte
Dim Xx As Word
'LM75
Dim Lm75read As Byte
'&H91/&H93 adres lezen
Dim Lm75write As Byte
'&H90/&H92 adres schrijven
Dim Temp_vk As Integer
'before comma value
Dim Temp_nk As Byte
'after comma value
Dim Msb As Byte
'Temperature for comma as Integer
-32768 tot +32767
Dim Lsb As Byte
Dim Vz As Byte 'voorteken (-/+)
Dim Btemp_vk As Byte
Const W5100_s3_rx_base =
&H7800
Const W5100_s2_rx_base = &H7000
Const W5100_s1_rx_base = &H6800
Const W5100_s0_rx_base = &H6000
Const W5100_s3_tx_base = &H5800
Const W5100_s2_tx_base = &H5000
Const W5100_s1_tx_base = &H4800
Const W5100_s0_tx_base = &H4000
Const W5100_sn_rx_mask = &H07FF
Const W5100_sn_tx_mask = &H07FF
'Used Wiz5100 ports and pins
Wiz5100_cs Alias Portb.2 ' Chipselect WIZ810MJ
'Used ports and pins
Config Wiz5100_cs = Output
' 0 = long board
' 1 = sandwichboard
Const Board = 1
Print "Start.."
Wait 1
#if Board 'sandwichboard
Wiz5100_res Alias Portd.3 'reset of WIZ810MJ
'Other used ports and pins
'Relais1 Alias Portd.4
'Relais2 Alias Portd.5
'Relais3 Alias Portd.6
'Relais4 Alias Portd.7
#elseif
Wiz5100_res Alias Portc.0 'long board
'Relais1 Alias Portc.1
'Relais2 Alias Portc.2
'Relais3 Alias Portc.3
'Relais4 Alias Portc.4
#endif
Config Wiz5100_res = Output
'I2c
Config Sda = Portb.7
Config Scl = Portb.6
I2cinit
'Configuration of the SPI-bus
Config Spi = Hard , Interrupt = Off , Data Order = Msb , Master = Yes ,
Polarity = Low , Phase = 0 , Clockrate = 4 , Noss = 0
'Init the spi pins
Spiinit
Wait 1
'Here we declare the used sub
routines
Declare Sub Wiz5100_init
Declare Sub Wiz5100_readvalue(byval Reg As Word)
Declare Sub Wiz5100_writevalue(byval Reg As Word , Byval Value As Byte)
Declare Sub Wiz5100_reset
Declare Sub Wiz5100_reply
'LM75
Declare Sub Dec_in_2er
Declare Sub 2er_in_dec
Declare Sub Read_temp
Declare Sub Resetpointer
Declare Sub Check
Declare Sub Readtemperature
Dim Wiz5100_opcode_read As
Byte
Wiz5100_opcode_read = 15
Dim Wiz5100_opcode_write As Byte
Wiz5100_opcode_write = 240
Wait 1
Call Wiz5100_init ' We
initialize the Wiz810MJ
'Assign all 2k memory to every socket for RX
Call Wiz5100_writevalue(w5100_rmsr , &B01010101) '
'Assign all 2k memory to every socket for TX
Call Wiz5100_writevalue(w5100_tmsr , &B01010101)
'protocol = UDP
Call Wiz5100_writevalue(w5100_s0_mr , Sn_mr_udp)
Call Wiz5100_writevalue(w5100_s0_port0 , &H00) ' port 161
Call Wiz5100_writevalue(w5100_s0_port1 , &HA1)
'give the open command
Call Wiz5100_writevalue(w5100_s0_cr , Sn_cr_open)
'wait for socket to come online
Do
Call Wiz5100_readvalue(w5100_s0_sr)
Loop Until Value = Sock_udp
'check on RECV
Do
Call Wiz5100_readvalue(w5100_s0_ir)
If Value.2 = 1 Then
Call Wiz5100_reply
Call Wiz5100_writevalue(w5100_s0_cr , Sn_cr_recv)
'reset interrupt
Call Wiz5100_writevalue(w5100_s0_ir , &B100)
Call Wiz5100_readvalue(w5100_s0_cr)
End If
Loop
End
Sub Wiz5100_init
Call Wiz5100_reset 'Hardware reset
'Register reset
Call Wiz5100_writevalue(w5100_mr , &H80)
'Set gateway IP adress
Call Wiz5100_writevalue(w5100_gar0 , 192)
'My gateway
Call Wiz5100_writevalue(w5100_gar1 , 168)
Call Wiz5100_writevalue(w5100_gar2 , 0)
Call Wiz5100_writevalue(w5100_gar3 , 1)
'Set Subnetmask
Call Wiz5100_writevalue(w5100_subr0 , 255)
'My networkmask
Call Wiz5100_writevalue(w5100_subr1 , 255)
Call Wiz5100_writevalue(w5100_subr2 , 255)
Call Wiz5100_writevalue(w5100_subr3 , 0)
'Set MAC
Call Wiz5100_writevalue(w5100_shar0
, &H00)
'My MAC-address
Call Wiz5100_writevalue(w5100_shar1 , &H10)
Call Wiz5100_writevalue(w5100_shar2 , &H20)
Call Wiz5100_writevalue(w5100_shar3 , &H30)
Call Wiz5100_writevalue(w5100_shar4 , &H40)
Call Wiz5100_writevalue(w5100_shar5 , &H50)
'Set own IP adress
Call Wiz5100_writevalue(w5100_sipr0 , 192)
'My IP-number
Call Wiz5100_writevalue(w5100_sipr1 , 168)
Call Wiz5100_writevalue(w5100_sipr2 , 0)
Call Wiz5100_writevalue(w5100_sipr3 , 50)
End Sub
Sub Wiz5100_readvalue(reg)
Adres = Reg
Reset Wiz5100_cs
Spiout Wiz5100_opcode_read , 1
Spiout Adresh , 1
Spiout Adresl , 1
Spiin Value , 1
Set Wiz5100_cs
End Sub
Sub Wiz5100_writevalue(reg ,
Value )
Adres = Reg
Reset Wiz5100_cs
Spiout Wiz5100_opcode_write , 1
Spiout Adresh , 1
Spiout Adresl , 1
Spiout Value , 1
Set Wiz5100_cs
End Sub
Sub Wiz5100_reset
Waitms 250
Wiz5100_res = 1
Waitms 250
Wiz5100_res = 0
Waitms 250
Wiz5100_res = 1
End Sub
Sub Wiz5100_reply
'What do we have to know?
'What is the IP-number of the
requester
Call Wiz5100_readvalue(&H6000)
Src_ip(1) = Value
Call Wiz5100_readvalue(&H6001)
Src_ip(2) = Value
Call Wiz5100_readvalue(&H6002)
Src_ip(3) = Value
Call Wiz5100_readvalue(&H6003)
Src_ip(4) = Value
Print "Source IP " ; Src_ip(1)
; "." ; Src_ip(2) ; "." ; Src_ip(3) ; "." ; Src_ip(4)
'What is the returnport of
the requester
Call Wiz5100_readvalue(&H6004)
Src_prt(1) = Value
Call Wiz5100_readvalue(&H6005)
Src_prt(2) = Value
Print "Source poort ";
Wsrc_prt = Src_prt(1)
Shift Wsrc_prt , Left , 8
Wsrc_prt = Wsrc_prt + Src_prt(2)
Print Wsrc_prt
'What is the length of the
UDP-packet
Call Wiz5100_readvalue(&H6006)
Length(1) = Value
Call Wiz5100_readvalue(&H6007)
Length(2) = Value
Wlength = Length(1)
Shift Wlength , Left , 8
Wlength = Wlength + Length(2)
Print "Length UDP-packet ";
Print Wlength
Print "version"
Call Wiz5100_readvalue(&H600a)
Print Hex(value)
Call Wiz5100_readvalue(&H600b)
Print Hex(value)
Call Wiz5100_readvalue(&H600c)
Print Hex(value)
'skip &H600d
'skip &H600e
'What is the community string?
public
For Xx = &H600F To &H6014
Call Wiz5100_readvalue(xx)
Print Chr(value)
Next Xx
Print "PDU-type"
Call Wiz5100_readvalue(&H6015)
Print Hex(value)
Call Wiz5100_readvalue(&H6016)
Print Hex(value)
Print "request ID"
Call Wiz5100_readvalue(&H6017)
Print Hex(value)
Call Wiz5100_readvalue(&H6018)
Print
Hex(value)
Call Wiz5100_readvalue(&H6019)
Print Hex(value)
Reqid(1) = Value
Call Wiz5100_readvalue(&H601a)
Print Hex(value)
Reqid(2) = Value
Print "error status"
Call Wiz5100_readvalue(&H601b)
Print Hex(value)
Call Wiz5100_readvalue(&H601c)
Print Hex(value)
Call Wiz5100_readvalue(&H601d)
Print Hex(value)
Print "error index"
Call Wiz5100_readvalue(&H601e)
Print Hex(value)
Call Wiz5100_readvalue(&H601f)
Print Hex(value)
Call Wiz5100_readvalue(&H6020)
Print Hex(value)
'skip &H6021
'skip &H6022
Print "Object identifier"
Call Wiz5100_readvalue(&H6023)
Print Hex(value)
Call Wiz5100_readvalue(&H6024)
Print Hex(value)
Call Wiz5100_readvalue(&H6025)
Print Hex(value)
Call Wiz5100_readvalue(&H6026)
Print Hex(value)
Call Wiz5100_readvalue(&H6027)
Print Hex(value)
Call Wiz5100_readvalue(&H6028)
Print Hex(value)
Call Wiz5100_readvalue(&H6029)
Print Hex(value)
Call Wiz5100_readvalue(&H602a)
Print Hex(value)
Call Wiz5100_readvalue(&H602b)
Print Hex(value)
Call Wiz5100_readvalue(&H602c)
Print Hex(value)
Call Wiz5100_readvalue(&H602d)
Print Hex(value)
Call Wiz5100_readvalue(&H602e)
Print Hex(value)
Print "Value"
Call Wiz5100_readvalue(&H602f)
Print Hex(value)
Call Wiz5100_readvalue(&H6030)
Print Hex(value)
'we are going to send something back...
'protocol = UDP
Call Wiz5100_writevalue(w5100_s0_mr , Sn_mr_udp)
Call Wiz5100_writevalue(w5100_s0_port0 , &H00)
'from port 161
Call Wiz5100_writevalue(w5100_s0_port1 , &HA1)
'give the open command
Call Wiz5100_writevalue(w5100_s0_cr , Sn_cr_open)
'wait for socket to come
online
Do
Call Wiz5100_readvalue(w5100_s0_sr)
Loop Until Value = Sock_udp
' IP-number from requester.....
Call Wiz5100_writevalue(w5100_s0_dipr0 , Src_ip(1))
Call Wiz5100_writevalue(w5100_s0_dipr1 , Src_ip(2))
Call Wiz5100_writevalue(w5100_s0_dipr2 , Src_ip(3))
Call Wiz5100_writevalue(w5100_s0_dipr3 , Src_ip(4))
'Destination port
Call Wiz5100_writevalue(w5100_s0_dport0 , Src_prt(1)) 'returnport from
requester
Call Wiz5100_writevalue(w5100_s0_dport1 , Src_prt(2))
'SNMP
Call Wiz5100_writevalue(&H4000 , &H30)
Call Wiz5100_writevalue(&H4001 , &H82)
Call Wiz5100_writevalue(&H4002 , &H00)
Call Wiz5100_writevalue(&H4003
, &H33)
'how much characters are following
Call Wiz5100_writevalue(&H4004
, &H02)
Call Wiz5100_writevalue(&H4005 , &H01)
Call Wiz5100_writevalue(&H4006 , &H00)
Call Wiz5100_writevalue(&H4007
, &H04)
Call Wiz5100_writevalue(&H4008 , &H06)
Call Wiz5100_writevalue(&H4009
, &H70)
'community string 'public'
Call Wiz5100_writevalue(&H400a ,
&H75)
Call Wiz5100_writevalue(&H400b , &H62)
Call Wiz5100_writevalue(&H400c , &H6C)
Call Wiz5100_writevalue(&H400d , &H69)
Call Wiz5100_writevalue(&H400e , &H63)
Call Wiz5100_writevalue(&H400f
, &HA2)
Call Wiz5100_writevalue(&H4010 , &H82)
Call Wiz5100_writevalue(&H4011 , &H00)
Call Wiz5100_writevalue(&H4012 , &H24)
Call Wiz5100_writevalue(&H4013 , &H02)
Call Wiz5100_writevalue(&H4014 , &H02)
Call Wiz5100_writevalue(&H4015 , Reqid(1))
Call Wiz5100_writevalue(&H4016 , Reqid(2))
Call Wiz5100_writevalue(&H4017 , &H02)
Call Wiz5100_writevalue(&H4018 , &H01)
Call Wiz5100_writevalue(&H4019 , &H00)
Call Wiz5100_writevalue(&H401a , &H02)
Call Wiz5100_writevalue(&H401b , &H01)
Call Wiz5100_writevalue(&H401c , &H00)
Call Wiz5100_writevalue(&H401d , &H30)
Call Wiz5100_writevalue(&H401e , &H82)
Call Wiz5100_writevalue(&H401f , &H00)
Call Wiz5100_writevalue(&H4020
, &H16)
'how much characters following
Call Wiz5100_writevalue(&H4021 , &H30)
Call Wiz5100_writevalue(&H4022 , &H82)
Call Wiz5100_writevalue(&H4023 , &H00)
Call Wiz5100_writevalue(&H4024
, &H12)
'how much characters following
Call Wiz5100_writevalue(&H4025
, &H06)
Call Wiz5100_writevalue(&H4026 , &H0D)
Call Wiz5100_writevalue(&H4027 , &H2B)
Call Wiz5100_writevalue(&H4028 , &H06)
Call Wiz5100_writevalue(&H4029 , &H01)
Call Wiz5100_writevalue(&H402a , &H04)
Call Wiz5100_writevalue(&H402b , &H01)
Call Wiz5100_writevalue(&H402c , &H82)
Call Wiz5100_writevalue(&H402d , &H3E)
Call Wiz5100_writevalue(&H402e , &H01)
Call Wiz5100_writevalue(&H402f , &H01)
Call Wiz5100_writevalue(&H4030 , &H02)
Call Wiz5100_writevalue(&H4031 , &H01)
Call Wiz5100_writevalue(&H4032 , &H01)
Call Wiz5100_writevalue(&H4033 , &H00)
Call Readtemperature
Call Wiz5100_writevalue(&H4034
, &H42)
Call Wiz5100_writevalue(&H4035 , &H01)
Call Wiz5100_writevalue(&H4036 , Btemp_vk)
'temperature
'set size
Call Wiz5100_writevalue(w5100_s0_tx_wr0 , 0)
Call Wiz5100_writevalue(w5100_s0_tx_wr1 , &H37)
'send
Call Wiz5100_writevalue(w5100_s0_cr , Sn_cr_send)
Do
Call Wiz5100_readvalue(w5100_s0_cr)
Loop Until Value = 0
End Sub
'Subroutines for the LM75
temperature sensor
'Found this routine on the
Internet - thanks to the German publisher
'We only will be using the INT-part
'But if you have a LCD connected you can have the temperature
display more precise
Sub
Readtemperature
Lm75read = &H91
Lm75write = &H90
Call Read_temp
Print "Temperature 1 " ; Chr(vz) ; Str(temp_vk) ; "." ; Chr(temp_nk) ; "
C" '
Btemp_vk = Temp_vk
End Sub
Sub Read_temp
I2cstart 'put LM75 addres on the i2c bus
I2cwbyte Lm75read 'read Temp register
I2crbyte Msb , Ack 'before the dot
I2crbyte Lsb , Nack 'after the dot
I2cstop
Call 2er_in_dec
Call Check
Call Resetpointer
End Sub
' *** 2e complement in
decimaal ***
Sub 2er_in_dec
If Msb.7 = 0 Then 'Check if temp is postive Bit7 = 0
Temp_vk = Msb
Else 'else negative
Temp_vk = Not Msb 'ignore
End If
End Sub
' *** Decimal 2e complement
***
Sub Dec_in_2er
Msb = Not Msb 'invert
Msb = Msb + 1 'increment
End Sub
' *** check on I2C Bus error
***
Sub Resetpointer
If Err = 1 Then
Print "I²C Bus Error " ; Err
End If
I2cstart
I2cwbyte Lm75write
I2cwbyte &B00000000 , Nack
I2cstop
If Err = 1 Then
Print "Resetpointer - I²C Bus Error "
End If
End Sub
' ***
do some calculation ***
Sub Check
' *** check positive
***
If Msb.7 = 1 Then
Vz = " "
Temp_vk = Makeint(msb , &HFF)
Else
Temp_vk = Makeint(msb , &H00) ' positive
End If
' *** check
temp negative and 0,5 °C bit
set ***
If Lsb.7 = 1 And Msb.7 = 1 Then
Incr Temp_vk
'increment before the comma
End If
' ***
check on
temp <> 0 and positive
If Msb <> 0 And Msb.7 = 0 Then
Vz = "+"
'Put a + in front
End If
' ***
check if temp =
-0,5°C ***
If Msb = 255 And Lsb = 255 Then
'-0,5 ° degrees correction
Vz =
"-"
End If
' *** check
exact 0°C ***
If Msb = 0 And Lsb.7 = 0 Then
'on 0 ° C
Vz = " "
End If
' **
after comma ***
If Lsb.7 = 1 Then
'after the comma value = ,5 ?
Temp_nk = "5"
Else
Temp_nk = "0"
End If
End Sub
If you got Cacti installed you can also monitor
your equipment. Here the round-trip time for a PING to a Red Bokito on
my own small network. Search on the Internet for the True Ping templates.
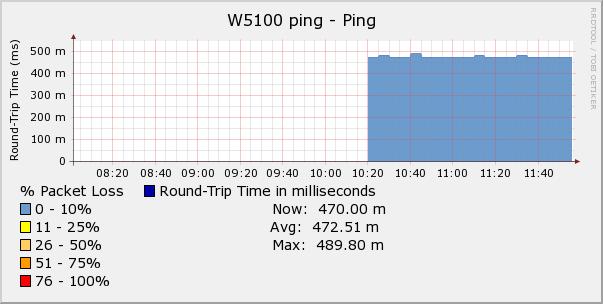
|
To be
continued.... (more sensors, and SNMP-trap) |
|
UDP-switch to be controlled by an iPhone UDP-remote
App |
With the iPhone app of
https://www.alcorn.com/products/UDP_Remote it would be nice to
control our long or short board.
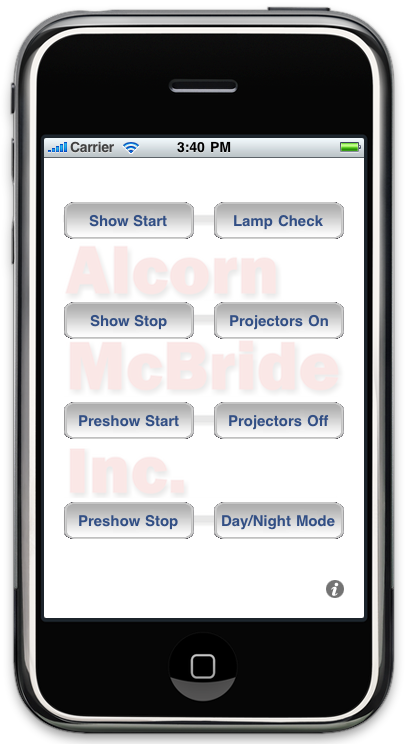
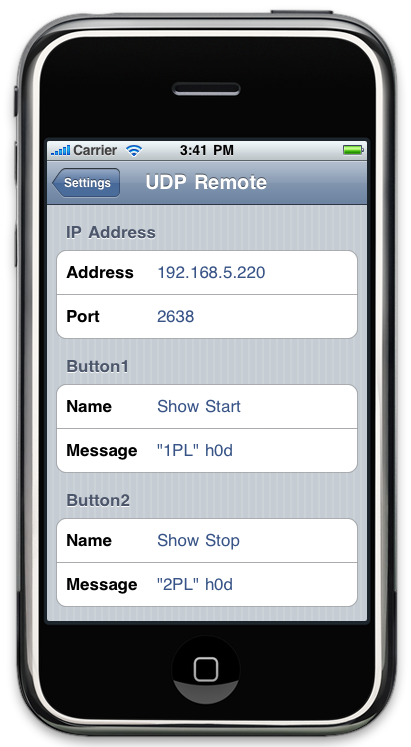
' UDP_Switch
'------------------------------------
' Atmega168 and WIZ810MJ - UDP_Switch
'------------------------------------
' Ben Zijlstra = 2011
'
' Hardware: WIZ810MJ long or sandwich board from https://benshobbycorner.nl/bzijlstra
$prog &HFF , &HE2 , &HD7 , &HF9
$regfile = "m168def.dat"
$crystal = 8000000
$baud = 9600
$hwstack = 128
$swstack = 128
$framesize = 128
$include "w5100.inc"
'EEPROM:
'001 = IP(1)
'002 = IP(2)
'003 = IP(3)
'004 = IP(4)
'005 = MAC(1)
'006 = MAC(2)
'007 = MAC(3)
'008 = MAC(4)
'009 = MAC(5)
'010 = MAC(6)
'011 = Netmask(1)
'012 = Netmask(2)
'013 = Netmask(3)
'014 = Netmask(4)
'015 = Gateway(1)
'016 = Gateway(2)
'017 = Gateway(3)
'018 = Gateway(4)
Dim Value As Byte
Dim Adres As Word
Dim Adresl As Byte At Adres Overlay
Dim Adresh As Byte At Adres + 1 Overlay
Dim Toets As Byte
Dim Mymac(6) As Byte
Dim Ippart(4) As String * 4
Dim Myip(4) As Byte
Dim Mygw(4) As Byte
Dim Mymsk(4) As Byte
Dim Mymacs(6) As String * 3
Dim Action As String * 12
Const W5100_s3_rx_base = &H7800
Const W5100_s2_rx_base = &H7000
Const W5100_s1_rx_base = &H6800
Const W5100_s0_rx_base = &H6000
Const W5100_s3_tx_base = &H5800
Const W5100_s2_tx_base = &H5000
Const W5100_s1_tx_base = &H4800
Const W5100_s0_tx_base = &H4000
Const W5100_sn_rx_mask = &H07FF
Const W5100_sn_tx_mask = &H07FF
Const User = "ADMIN"
Const Password = "FIRE"
'Used Wiz5100 ports and pins
Wiz5100_cs Alias Portb.2 ' Chipselect WIZ810MJ
Config Wiz5100_cs = Output
' 0 = long board
' 1 = sandwichboard
Const Board = 0
Print "Start.."
Wait 1
#if Board 'sandwichboard
Wiz5100_res Alias Portd.3 'reset of WIZ810MJ
'Other used ports and pins
Relais1 Alias Portd.4
Relais2 Alias Portd.5
Relais3 Alias Portd.6
Relais4 Alias Portd.7
#elseif
Wiz5100_res Alias Portc.0 'long board
Relais1 Alias Portc.1
Relais2 Alias Portc.2
Relais3 Alias Portc.3
Relais4 Alias Portc.4
#endif
Config Relais1 = Output
Config Relais2 = Output
Config Relais3 = Output
Config Relais4 = Output
Relais1 = 1
Relais2 = 1
Relais3 = 1
Relais4 = 1
Config Wiz5100_res = Output
'Configuration of the SPI-bus
Config Spi = Hard , Interrupt = Off , Data Order = Msb , Master = Yes ,
Polarity = Low , Phase = 0 , Clockrate = 4 , Noss = 0
'Init the spi pins
Spiinit
Wait 1
'Here we declare the used sub routines
Declare Sub Wiz5100_init
Declare Sub Wiz5100_readvalue(byval Reg As Word)
Declare Sub Wiz5100_writevalue(byval Reg As Word , Byval Value As Byte)
Declare Sub Wiz5100_reset
Declare Sub Banner
Declare Sub Read_ip
Declare Sub Read_gw
Declare Sub Read_mask
Declare Sub Read_mac
Declare Sub Configure
Declare Sub Print_ip
Declare Sub Print_mask
Declare Sub Print_gw
Declare Sub Print_mac
Declare Sub Take_action
Dim Wiz5100_opcode_read As Byte
Wiz5100_opcode_read = 15
Dim Wiz5100_opcode_write As Byte
Wiz5100_opcode_write = 240
Wait 1
Call Banner
Print "Press key for configuration..."
Call Read_ip
Call Read_gw
Call Read_mask
Call Read_mac
Call Wiz5100_init ' We initialize the Wiz810MJ
'now open a UDP-socket
'Assign all 2k memory to every socket for RX
Call Wiz5100_writevalue(w5100_rmsr , &B01010101) '
'Assign all 2k memory to every socket for TX
Call Wiz5100_writevalue(w5100_tmsr , &B01010101)
'protocol = UDP
Call Wiz5100_writevalue(w5100_s0_mr , Sn_mr_udp)
Call Wiz5100_writevalue(w5100_s0_port0 , &H13) ' port 5000
Call Wiz5100_writevalue(w5100_s0_port1 , &H88)
'give the open command
Call Wiz5100_writevalue(w5100_s0_cr , Sn_cr_open)
'wait for socket to come online
Do
Call Wiz5100_readvalue(w5100_s0_sr)
Loop Until Value = Sock_udp
'check on RECV
Do
Toets = Ischarwaiting()
If Toets = 1 Then
Toets = Waitkey()
Call Configure
End If
Action = ""
Call Wiz5100_readvalue(w5100_s0_ir)
If Value.2 = 1 Then
Call Wiz5100_readvalue(&H6008)
Action = Chr(value)
Call Wiz5100_readvalue(&H6009)
Action = Action + Chr(value)
Call Wiz5100_readvalue(&H600a)
Action = Action + Chr(value)
Call Wiz5100_readvalue(&H600b)
Action = Action + Chr(value)
Call Wiz5100_readvalue(&H600c)
Action = Action + Chr(value)
Call Wiz5100_readvalue(&H600d)
Action = Action + Chr(value)
Call Wiz5100_readvalue(&H600e)
Action = Action + Chr(value)
Call Wiz5100_readvalue(&H600f)
Action = Action + Chr(value)
Call Wiz5100_readvalue(&H6010)
Action = Action + Chr(value)
Call Wiz5100_readvalue(&H6011)
Action = Action + Chr(value)
Call Wiz5100_readvalue(&H6012)
Action = Action + Chr(value)
'in action the actionstring
Call Take_action
Call Wiz5100_writevalue(w5100_s0_cr , Sn_cr_recv)
'reset interrupt
Call Wiz5100_writevalue(w5100_s0_ir , &B100)
Call Wiz5100_readvalue(w5100_s0_cr)
End If
Loop
End
Sub Wiz5100_init
Call Wiz5100_reset 'Hardware reset
'Register reset
Call Wiz5100_writevalue(w5100_mr , &H80)
'Set gateway IP adress
Call Wiz5100_writevalue(w5100_gar0 , Mygw(1)) 'My gateway
Call Wiz5100_writevalue(w5100_gar1 , Mygw(2))
Call Wiz5100_writevalue(w5100_gar2 , Mygw(3))
Call Wiz5100_writevalue(w5100_gar3 , Mygw(4))
'Set Subnetmask
Call Wiz5100_writevalue(w5100_subr0 , Mymsk(1)) 'My networkmask
Call Wiz5100_writevalue(w5100_subr1 , Mymsk(2))
Call Wiz5100_writevalue(w5100_subr2 , Mymsk(3))
Call Wiz5100_writevalue(w5100_subr3 , Mymsk(4))
'Set MAC
Call Wiz5100_writevalue(w5100_shar0 , Mymac(1)) 'My MAC-address
Call Wiz5100_writevalue(w5100_shar1 , Mymac(2))
Call Wiz5100_writevalue(w5100_shar2 , Mymac(3))
Call Wiz5100_writevalue(w5100_shar3 , Mymac(4))
Call Wiz5100_writevalue(w5100_shar4 , Mymac(5))
Call Wiz5100_writevalue(w5100_shar5 , Mymac(6))
'Set own IP adress
Call Wiz5100_writevalue(w5100_sipr0 , Myip(1)) 'My IP-number
Call Wiz5100_writevalue(w5100_sipr1 , Myip(2))
Call Wiz5100_writevalue(w5100_sipr2 , Myip(3))
Call Wiz5100_writevalue(w5100_sipr3 , Myip(4))
End Sub
Sub Wiz5100_readvalue(reg)
Adres = Reg
Reset Wiz5100_cs
Spiout Wiz5100_opcode_read , 1
Spiout Adresh , 1
Spiout Adresl , 1
Spiin Value , 1
Set Wiz5100_cs
End Sub
Sub Wiz5100_writevalue(reg , Value )
Adres = Reg
Reset Wiz5100_cs
Spiout Wiz5100_opcode_write , 1
Spiout Adresh , 1
Spiout Adresl , 1
Spiout Value , 1
Set Wiz5100_cs
End Sub
Sub Wiz5100_reset
Waitms 250
Wiz5100_res = 1
Waitms 250
Wiz5100_res = 0
Waitms 250
Wiz5100_res = 1
End Sub
' Routine to get a configuration-screen on the RS232 port
'
Sub Configure
Stop Watchdog
Local Hulp1 As Byte
Local Hulp2 As Byte
Local Hulp3 As Word
Local Hulp4 As Byte
Local Yn As String * 1
Local Ipnr As String * 15
Local Dot1 As Byte
Local Dot2 As Byte
Local Dot3 As Byte
Local Dot4 As Byte
Local Dot5 As Byte
Local Iptemp As String * 1
Local Bfr As String * 20
Call Read_ip
Call Read_gw
Call Read_mask
Call Read_mac
Do
Call Banner
Print
Input "Login: " , Bfr
If Left(bfr , 5) = User Then
Print
Input "Password: " , Bfr
End If
Loop Until Left(bfr , 4) = Password
Call Banner
Print "Configure mode:"
Print
Print "Network:"
Print
Print "IP-number: ";
Call Print_ip
Input "Change IP-number (y/n) " , Yn
If Yn = "y" Then
Print
Print "Input IP-numbers as decimals, seperated by a dot (like
192.168.1.106)"
Print
Input Ipnr
Hulp1 = Split(ipnr , Ippart(1) , ".")
Myip(1) = Val(ippart(1))
Myip(2) = Val(ippart(2))
Myip(3) = Val(ippart(3))
Myip(4) = Val(ippart(4))
Print
Print "New IP-number: ";
Call Print_ip
Print
Input "Accept IP-number (y/n) " , Yn
If Yn = "y" Then
Writeeeprom Myip(1) , 1 : Writeeeprom Myip(2) , 2
Writeeeprom Myip(3) , 3 : Writeeeprom Myip(4) , 4
End If
Print
Print "IP-number: ";
Call Read_ip
Call Print_ip
End If
Print
'Netmask
Print "NetMask: ";
Call Print_mask
Input "Change NetMask (y/n) " , Yn
If Yn = "y" Then
Print
Print "Input IP-numbers as decimals, seperated by a dot"
Print
Input Ipnr
Hulp1 = Split(ipnr , Ippart(1) , ".")
Mymsk(1) = Val(ippart(1))
Mymsk(2) = Val(ippart(2))
Mymsk(3) = Val(ippart(3))
Mymsk(4) = Val(ippart(4))
Print
Print "New Mask: ";
Call Print_mask
Print
Input "Accept NetMask (y/n) " , Yn
If Yn = "y" Then
Writeeeprom Mymsk(1) , 11 : Writeeeprom Mymsk(2) , 12
Writeeeprom Mymsk(3) , 13 : Writeeeprom Mymsk(4) , 14
End If
Print
Print "NetMask: ";
Call Read_mask
Call Print_mask
End If
Print
'Gateway
Print "Gateway: ";
Call Print_gw
Input "Change Gateway (y/n) " , Yn
If Yn = "y" Then
Print
Print "Input IP-numbers as decimals, seperated by a dot"
Print
Input Ipnr
Hulp1 = Split(ipnr , Ippart(1) , ".")
Mygw(1) = Val(ippart(1))
Mygw(2) = Val(ippart(2))
Mygw(3) = Val(ippart(3))
Mygw(4) = Val(ippart(4))
Print
Print "New Gateway: ";
Call Print_gw
Print
Input "Accept Gateway (y/n) " , Yn
If Yn = "y" Then
Writeeeprom Mygw(1) , 15 : Writeeeprom Mygw(2) , 16
Writeeeprom Mygw(3) , 17 : Writeeeprom Mygw(4) , 18
End If
Print
Print "Gateway: ";
Call Read_gw
Call Print_gw
End If
Print
Print "MAC-Address: ";
Call Read_mac
Call Print_mac
Input "Change MAC-address (y/n) " , Yn
If Yn = "y" Then
Mac:
Print
Print "Input MAC-address in hexadecimals (like 00-34-35-36-37-48)"
Print
Input Bfr
If Len(bfr) <> 17 Then Goto Mac
Hulp1 = Split(bfr , Mymacs(1) , "-")
Mymac(1) = Hexval(mymacs(1))
Mymac(2) = Hexval(mymacs(2))
Mymac(3) = Hexval(mymacs(3))
Mymac(4) = Hexval(mymacs(4))
Mymac(5) = Hexval(mymacs(5))
Mymac(6) = Hexval(mymacs(6))
Print
Print "New MAC-address: ";
Call Print_mac
'Print
Input "Accept MAC-address (y/n) " , Yn
If Yn = "y" Then
Writeeeprom Mymac(1) , 5 : Writeeeprom Mymac(2) , 6
Writeeeprom Mymac(3) , 7 : Writeeeprom Mymac(4) , 8
Writeeeprom Mymac(5) , 9 : Writeeeprom Mymac(6) , 10
End If
Print
Print "MAC-address: ";
Call Read_mac
Call Print_mac
End If
Summary:
Print Chr(&H1b) ; "[2J"; ' ANSI goto 0/0
Print "Summary:"
Print
Print "Network:"
Print
Print "IP-number : " ; Myip(1) ; "." ; Myip(2) ; "." ; Myip(3) ; "." ;
Myip(4)
Print
Print "NetMask : " ; Mymsk(1) ; "." ; Mymsk(2) ; "." ; Mymsk(3) ; "." ;
Mymsk(4)
Print
Print "Gateway : " ; Mygw(1) ; "." ; Mygw(2) ; "." ; Mygw(3) ; "." ;
Mygw(4)
Print
Print "Mac-address : " ; Hex(mymac(1)) ; "-" ; Hex(mymac(2)) ; "-" ; Hex(mymac(3))
; "-" ; Hex(mymac(4)) ; "-" ; Hex(mymac(5)) ; "-" ; Hex(mymac(6))
Print
Print "Rebooting"
Wait 4
Goto 0000
End Sub
'Read IP-number from Eeprom
'
Sub Read_ip
Readeeprom Myip(1) , 1 : Readeeprom Myip(2) , 2
Readeeprom Myip(3) , 3 : Readeeprom Myip(4) , 4
End Sub
' Routine to print the IP-number
'
Sub Print_ip
Print Myip(1) ; "." ; Myip(2) ; "." ; Myip(3) ; "." ; Myip(4)
End Sub
' Routine to read the netmask from the EEPROM
'
Sub Read_mask
Readeeprom Mymsk(1) , 11 : Readeeprom Mymsk(2) , 12
Readeeprom Mymsk(3) , 13 : Readeeprom Mymsk(4) , 14
End Sub
' Routine to print the netmask
'
Sub Print_mask
Print Mymsk(1) ; "." ; Mymsk(2) ; "." ; Mymsk(3) ; "." ; Mymsk(4)
End Sub
' Routine to read the gateway from the EEPROM
'
Sub Read_gw
Readeeprom Mygw(1) , 15 : Readeeprom Mygw(2) , 16
Readeeprom Mygw(3) , 17 : Readeeprom Mygw(4) , 18
End Sub
' Routine to print the gateway
'
Sub Print_gw
Print Mygw(1) ; "." ; Mygw(2) ; "." ; Mygw(3) ; "." ; Mygw(4)
End Sub
' Routine to read the MAC-address from the EEPROM
'
Sub Read_mac
Readeeprom Mymac(1) , 5 : Readeeprom Mymac(2) , 6
Readeeprom Mymac(3) , 7 : Readeeprom Mymac(4) , 8
Readeeprom Mymac(5) , 9 : Readeeprom Mymac(6) , 10
End Sub
' Routine to print the MAC-address
'
Sub Print_mac
Print Hex(mymac(1)) ; "-" ; Hex(mymac(2)) ; "-" ; Hex(mymac(3)) ; "-" ;
Hex(mymac(4)) ; "-" ; Hex(mymac(5)) ; "-" ; Hex(mymac(6))
End Sub
' Routine to print a banner
'
Sub Banner
Print Chr(&H1b) ; "[2J"; ' ANSI goto 0/0
Print "UDP-Switch - configuration-menu - ver. 1.0"
Print
Print
End Sub
Sub Take_action
Print Action
Select Case Action
Case "relais1= on" : Relais1 = 0
Case "relais1=off" : Relais1 = 1
Case "relais2= on" : Relais2 = 0
Case "relais2=off" : Relais2 = 1
Case "relais3= on" : Relais3 = 0
Case "relais3=off" : Relais3 = 1
Case "relais4= on" : Relais4 = 0
Case "relais4=off" : Relais4 = 1
End Select
'put all memory pointers back on &H4000 and &H6000
Call Wiz5100_writevalue(w5100_s0_mr , Sn_mr_udp) ' protocol UDP
Call Wiz5100_writevalue(w5100_s0_port0 , &H13) ' port 5000
Call Wiz5100_writevalue(w5100_s0_port1 , &H88)
'give the open command
Call Wiz5100_writevalue(w5100_s0_cr , Sn_cr_open)
'wait for socket to come online
Do
Call Wiz5100_readvalue(w5100_s0_sr)
Loop Until Value = Sock_udp
End Sub In the iPhone at
settings for the UDP_Remote, you can give the 8 buttons any name and the
commands that are put under the buttons are:
"relais1= on"
"relais1=off"
"relais2= on"
"relais2=off"
"relais3= on"
"relais3=off"
"relais4= on"
"relais5=off" I liked to get the strings
all the same size so there is a space before on |
Putting
the hardware together |
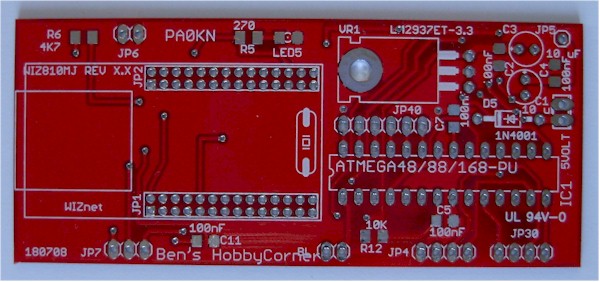
Step 1. A bare Small
board.
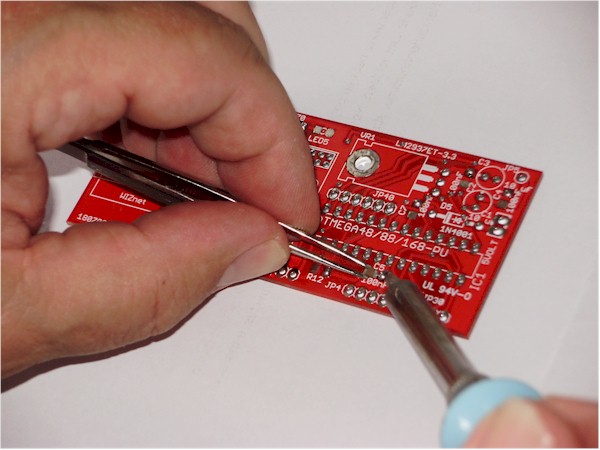
Step 2. SMD first.
The way I do this. Put
on one of the SMD sides on the PCB a small piece of tin.
Take a pair of tweezers, and slide the SMD part into the melting tin.
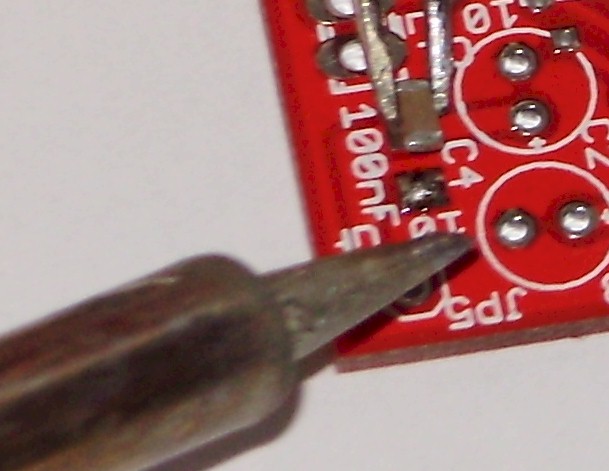
Step 3. Sliding the SMD
part.
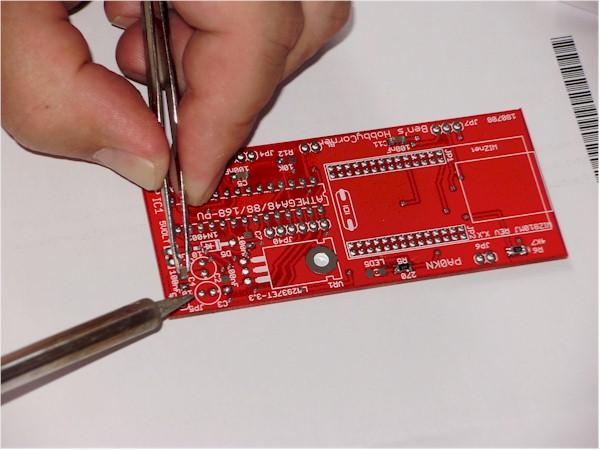
Step 4. Only 9 SMD parts.
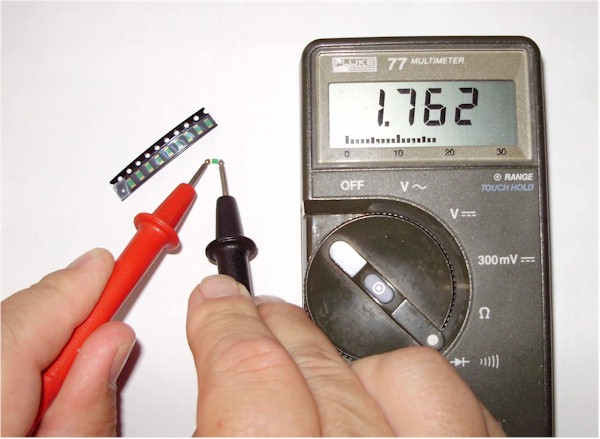
Step 5. SMD LED, which
direction?
Put your multimeter on
rectifier and check the LED. It should burn when connected right.
Right side is the cathode (on picture and on the PCB).
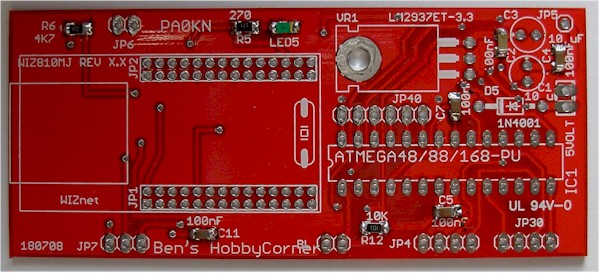
Step 6. SMD ready. 270, 4K7,
10K, 100 nF, LED.
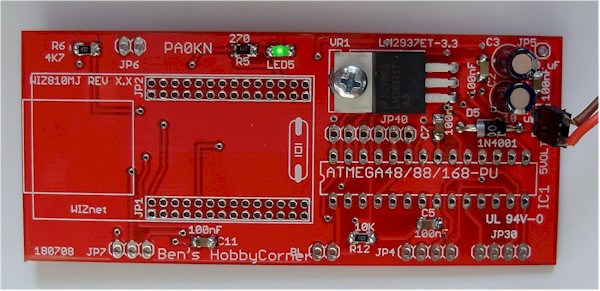
Step 7. Regulator
LM2937-3,3, diode IN4001, elcos 10 uF and the header for the power.
If you put power on it
you will know that the LED is put on the right way.
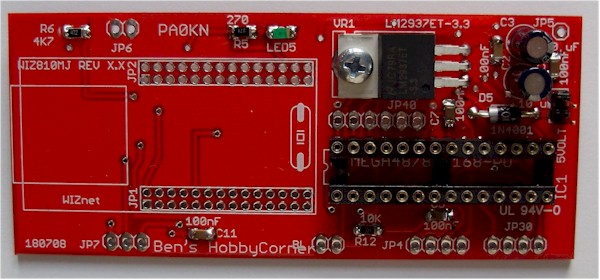
Step 8. The socket for
the Atmega168.
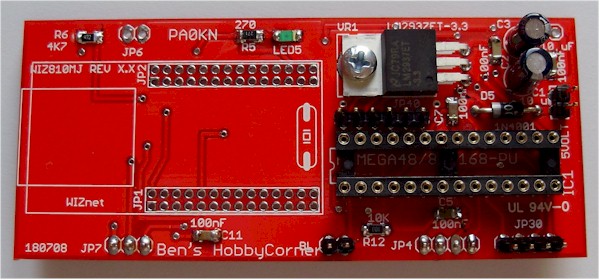
Step 9. Headers.
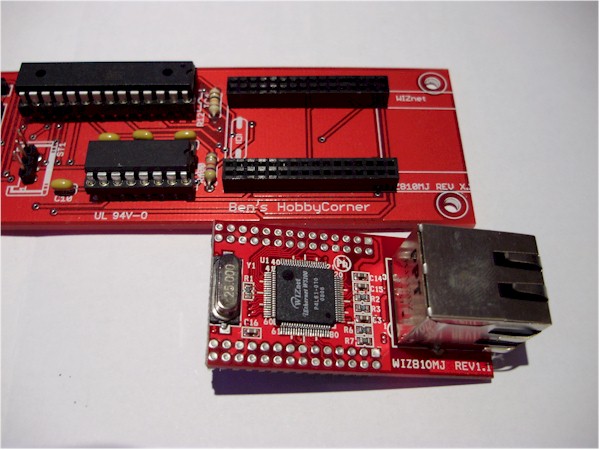
Step 10. Ready to put the
WIZ810MJ-module in its place.
Every module I sent has been tested on my test board.
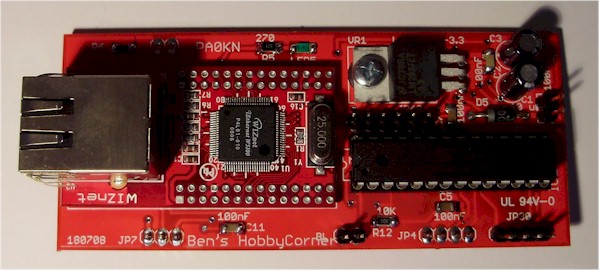
Step 11. WIZ810MJ and
microcontroller mounted.
Put the PING-test from
the begin of this tutorial in the microcontroller, take care of the fuse bits.
Here the hex-file and
the source of the PING-program.
WIZ810MJ_PING_SMALL.HEX
WIZ810MJ_PING_LONG.HEX
Wiz810mj_ping.bas
This way you switch
between long and small board
' 0 = long board
' 1 = sandwichboard
Const Board = 0
Step 12. Put a network cable
in the WIZ810MJ and put power on the board.
Start a PING <ip-number of your wiznet-module> -t on your PC and if everything is
oke you will see this.
In the background a ARP-request is done.
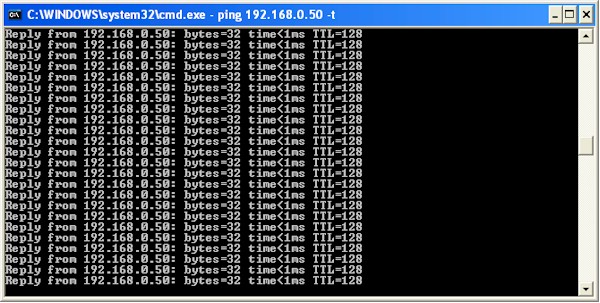
What you should see.....
Till now, only one board
didn't work right away. The 4K7 SMD didn't make good contact so the
WIZ810MJ-module wasn't put in SPI-Enable mode. Have put more than 30
boards together now.
Take care of the fuse bits
of the Atmega168. Standard they are sold with 1 MHz internal RC
Oscillator.
An 8 MHz Oscillator is divided by 8. You should put the lock and fuse bits
like this:
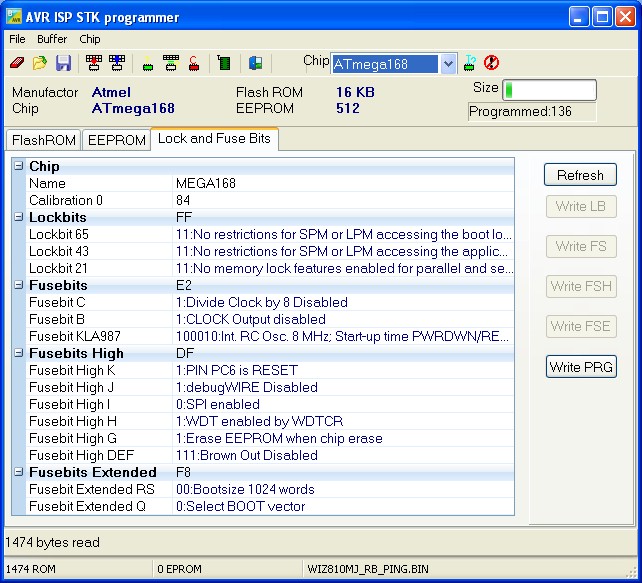
Later on you should put Fuse bit
High G on 0.
IP-number, Subnet mask and Gateway will be stored in EEPROM.
Not done yet, now the relays/RS232-board
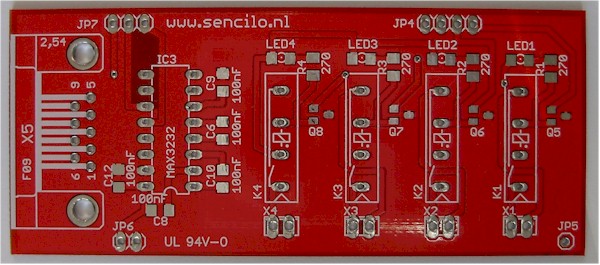
Step 10. Bare relays/RS232-board.
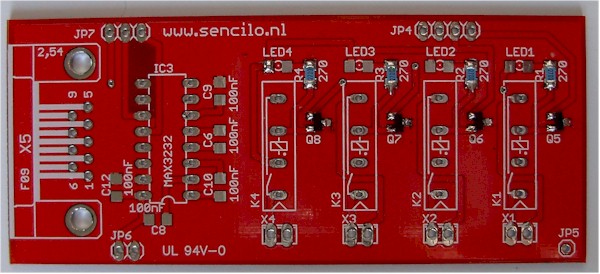
Step 11. SMD transistors
DTA143ET (PNP) and resistors 270 ohm.
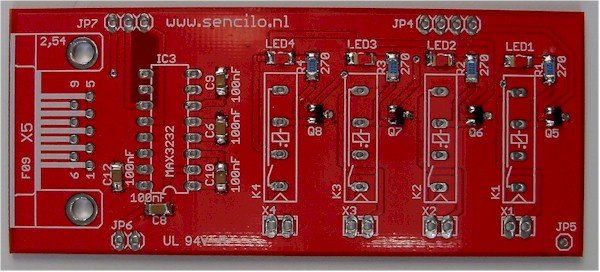
Step 12. 100 nF and LEDs.
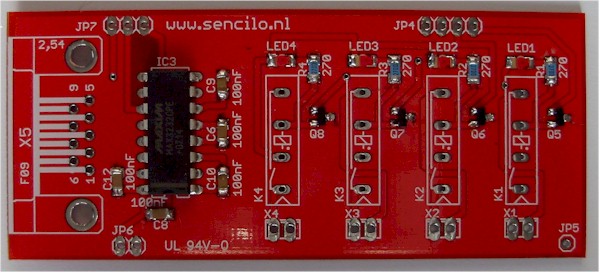
Step 13. Max3232.
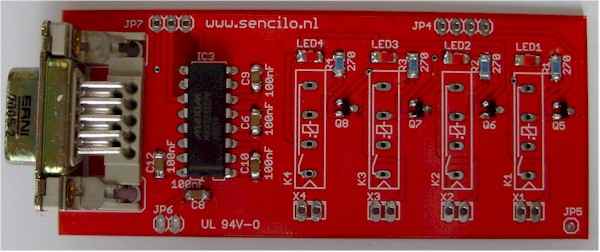
Step 14. SubD-9 female
connector.
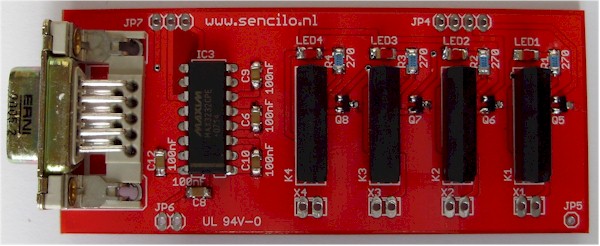
Step 15. Relays.
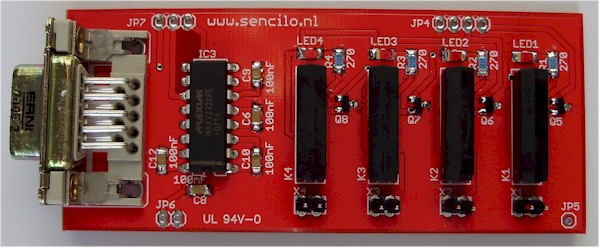
Step 16. Headers.
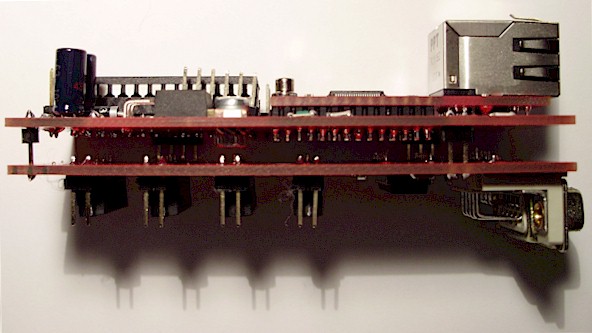
Step 17. The two boards
together.
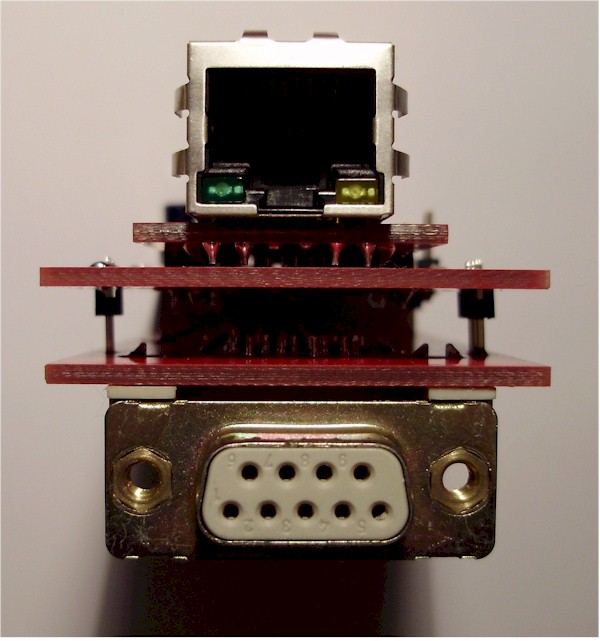
Ready.
The relay MEDER
SIL05-1A72-71D works at 5 volts. So 5 volts should be applied to the
power connector. The LM2937-3,3 brings the power to 3,3 volts for the
WIZ810MJ-module and the Atmega168 microcontroller. However if you want
to apply a higher voltage you can put a 78L05 on JP5 between the top and
the lower board. Then a maximum of 30 volt can be applied without any
trouble.
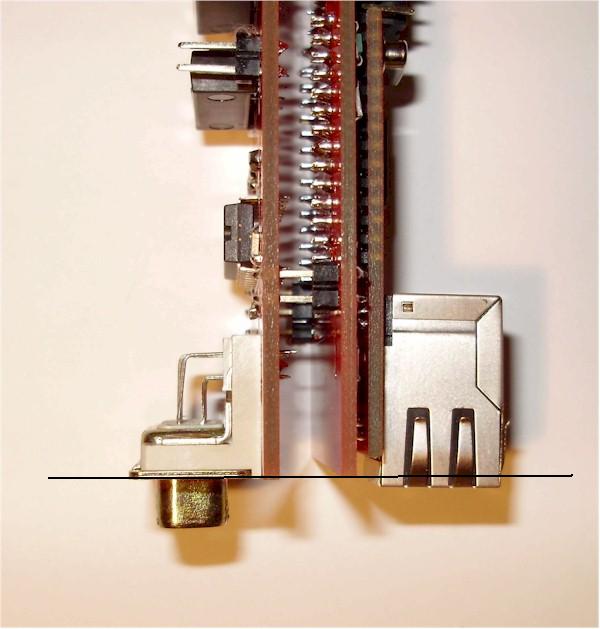
This way the module
could be mounted in its case.
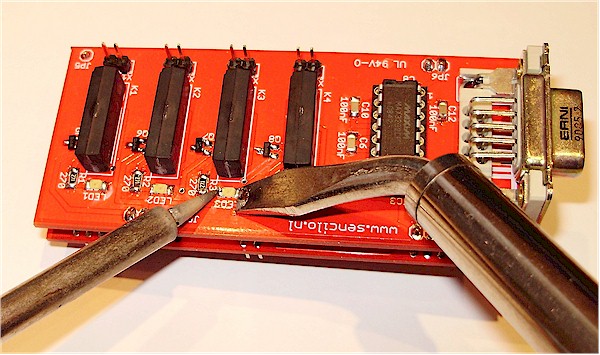
You got the LEDS the
wrong way on the board, here a trick to remove them with two irons.
|
Long
way, but at the end we get this... |
Small sandwichboard or
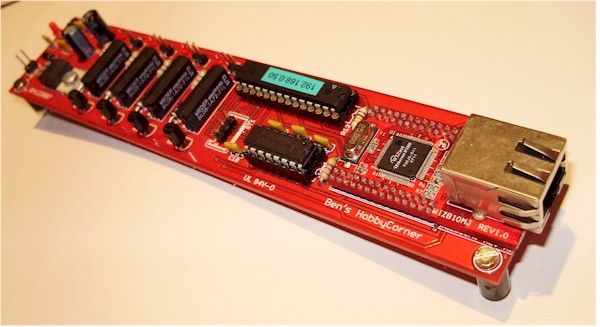 long
web server with four relays
Or a web server with up to eight I2c-LM75 temperature sensors
and four relays
Here some more
documentation of this design.
https://www.circuitcellar.com/wiznet/winners/DE/001141.html
|
Sandwichboard
used in EcoServer, here on display at the Cebit in Hannover |
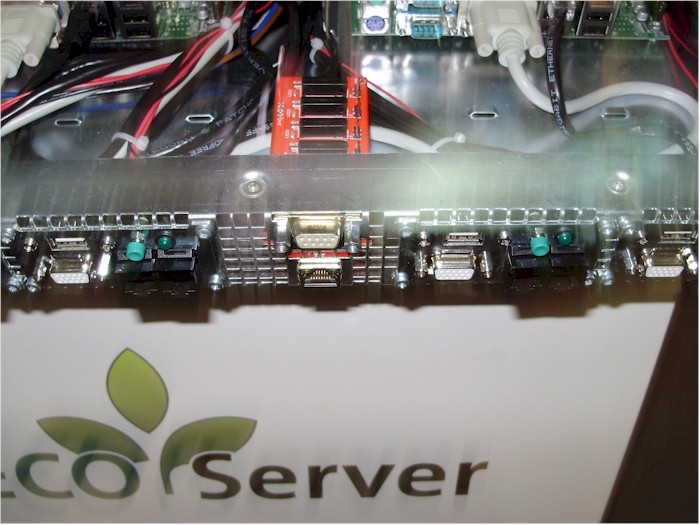
Check
for more info www.ecoserver.nl
|
Thanks
to: |

Thanks
to Mark Alberts
the creator of Bascom-AVR
www.mcselec.com
Bought my copy of Bascom-8051 in 1995
a few years later Bascom-AVR
Great stuff!!!

Thanks
to Thomas Heldt
He created the first Bascom-AVR-code for the WIZ810MJ
https://mikrocontroller.heldt.eu
(Held is hero in Dutch)

Thanks to Dietmar Steiner
He converted the WIZ810MJ code to work on the WIZ812MJ.
On Atmega8, 32, 64 and 644
Here
is his code
You
can reach Dietmar at
steiner(at)key-it.de

Thanks
to Fred Eady
With the Easy TCP/IP board from Fred I started
my survey on embedded Ethernet.
www.edtp.com
Where
are his splendid articles in Circuit Cellar?

Thanks
to Hugh Duff
for the Ethernet I/O Control Panel
www.drftech.com
Together we have build the AVR Ethernet I/O board
Flags can be downloaded at
www.3DFlags.com
|
Ben
Zijlstra - Ben's HobbyCorner - 2008 |
|